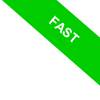
Python's endswith Method
In Python, the handy endswith() method helps you find out if a string ends with a specific substring. Here's how you use it:
string.endswith(substring, start, end)
Simply write the string's name, followed by the ".endswith()" method. Inside the parentheses, you can indicate:
- the substring you're looking for
- 'start' as the position in the string where the search begins
- 'end' as the position in the string where the search should stop
The method returns True if the string ends with the specified substring, and False otherwise.
Keep in mind that the first parameter is required, while 'start' and 'end' are optional. If you don't provide them, the method will check the entire string.
Let's see an example in action.
First, assign a string to a variable.
>>> string = "www.nigiara.com"
Now, use the endswith() method to check if the string ends with ".com".
Type the command string.endswith(".com")
>>> string.endswith(".com")
The method returns True because the string does indeed end with ".com".
True
Now, let's try string.endswith(".it")
>>> string.endswith(".it")
In this case, the method returns False because the string doesn't end with ".it.
False
Here's another real-life example.
Imagine you have a list of email addresses in the 'emails' variable.
emails = ["nigiara@gmail.com", "topolino@nigiara.com", "paperino@nigiara.com"]
Now, let's say you want to find only the emails that end with "@gmail.com".
You can use a 'for' loop, an 'if' statement, and the endswith() method to accomplish this:
- >>> for email in emails:
- if email.endswith("@gmail.com"):
- print(email)
This loop will iterate through all elements in the list and display only those that end with "@gmail.com":
nigiara@gmail.com
With this approach, you can quickly search for specific patterns within strings without the need to manipulate them.
You can also tailor the endswith() method by adding the 'start' and 'end' parameters to specify the character range for the substring search.
For instance, let's define a string:
>>> string = "www.nigiara.com"
Type string.endswith(".com", 13) to search for ".com" starting from position 13:
>>> string.endswith(".com", 13)
The method searches for the substring ".com" starting from position 13 in the string.
In this scenario, the method returns False because it doesn't find the substring at the end of the selected range:
False
Now, let's try string.endswith(".com", 1, 6)
>>> string.endswith(".com", 1,6)
The method searches for the substring ".com" from position 1 to position 6 in the string index.
Again, the method returns False because the substring isn't found at the end of the selected range:
False
Remember, Python's endswith() method is case-sensitive by default.. This means that if you pass an uppercase string as an argument, the method will only search for input strings that end with that uppercase string, ignoring those in lowercase. For example, define the string:
>>> string = "WWW.NIGIARA.COM"
Then search for the substring ".com"
>>> string.endswith(".com")
The method returns False because it distinguishes between lowercase and uppercase letters.
False
If you want the endswith() method to be case-insensitive, you can convert both the initial string and the argument passed to the method to either uppercase or lowercase before passing it to the method. For example, type this instruction:
>>> string.lower().endswith(".com")
This command first converts the string to lowercase using the lower() method and then searches for the substring ".com" at the end of the string with endswith(). In this case, the result is True.
True