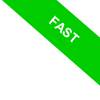
Python isdigit() Method
Have you ever wondered how to check if a string is solely made up of numbers in Python? Well, Python's got your back with a handy method called isdigit(). Here's how it works:
stringa.isdigit()
This method is tailor-made for String objects. Although it doesn't need any parameters, don't forget to add those parentheses. They're part of the party!
It'll give you a big thumbs-up (True) if the string is filled to the brim with numbers, and a shake of the head (False) if not.
Note. Here's a handy reminder: a string is considered full of numbers only if it's a sequence of characters that represent integers. So, "12345" would get a high-five for being all numbers, while "12.345" would get a polite "nope".
Let's roll up our sleeves and dive into an example.
Start by defining a string with numeric characters
>>> number = "12345"
Then, use the isdigit() method to check if your string is a numbers-only club.
>>> number.isdigit()
Voila! The method returns True because our string "12345" is all about those digits.
True
Now, let's switch things up with another example.
Define a string with numeric characters and a decimal point.
>>> number = "123.45"
Use the same isdigit() method to see if it's all about the numbers.
>>> number.isdigit()
Oops! The method says False this time. Our string "123.45" snuck in a decimal point ".".
False
What's cool is, the isdigit() method can even recognize those fancy Unicode characters with superscript and subscript numbers.
>>> number = "2²"
>>> number.isdigit()
The isdigit() method says True. Nice!
True
Note. Just a heads-up though. While Unicode characters with superscript and subscript numbers are considered numeric, they aren't decimal. So, isdigit() welcomes them, but isdecimal() gives them the cold shoulder.
>>> number = "2²"
>>> number.isdecimal()
The isdecimal method isn't impressed. It returns False.
False
Python offers two more methods, isdecimal() and isnumeric(), to check if a string is number-laden.
But beware, these methods aren't identical triplets.
Take this script for example:
numero = "½"
print(numero.isdigit())
print(numero.isdecimal())
print(numero.isnumeric())
These three siblings give different responses.
False
False
True
While isdigit() and isdecimal() dismiss "½" as a non-number, isnumeric() gives it a nod of approval.