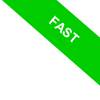
The rindex() Method in Python
The rindex() method in Python is a handy string method that finds the index of the last occurrence of a specific substring within a given string. Here's how it works:
string.rindex(substring[, start[, end]])
In this case, the "string" object is the one you want to search.
This method has three parameters:
- Substring: The substring you're looking for within the string.
- Start: The start is the index position from which to kick off the search. It's optional, with the default being 0 (the beginning of the string).
- End: The end is the index position where the search should wrap up. This is also optional, and the default is the last character of the string.
The method returns the index of the last occurrence of the substring within the string, searching from right to left.
If the method can't find the substring in the string, it won't return anything and will raise a ValueError exception with the message "substring not found."
Let's look at a practical example.
Suppose you have the following string assigned to the variable myVar:
>> myVar = "Mississippi"
To find the position of the substring "ssi" in the string's index using the rindex() method, you'd do this:
>> myVar.rindex("ssi")
The string "Mississippi" contains two occurrences of the substring "ssi." The first one starts at position 2, while the second one begins at position 5 of the index.
Since rindex() returns the position of the last occurrence of the word "ssi," you'll get the result 5.
5
So, in a nutshell, the rindex() method always provides the index position of the last occurrence of a word within a string.
If you're looking to find the position of the first occurrence of the substring instead, go ahead and use the index() method.