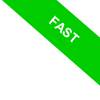
The ljust() Method in Python
The ljust() method in Python gives you a left-justified string, padded on the right side with a filler character until it reaches a certain length.
string.ljust(length[, filler])
Here, the string object is the string you want to align to the left.
This method accepts two parameters.
- The 'length' represents the total character count of the string after processing.
- The 'filler' (optional) is the character you want to use to pad the string to the right until it attains a certain total length.
The method outputs a string of the length you've specified, with the original text left-aligned and a sequence of filler characters on the right.
Note. The filler character can also be a character sequence. If you don't specify it, a blank space is used by default.
Now, let's try a little example.
We'll start with a simple string assigned to 'text':
>>> text = "Hello"
Now, let's say we want a 10-character string, with "Hello" snug over on the left side.
And for fun, we'll fill the remaining space with asterisks:
>>> text.ljust(10, "*")
What do we get? A neat 10-character string, with "Hello" on the left and a bunch of asterisks taking up the rest of the space.
Hello*****
In this way, you can format a string to a predefined length, filling up the right side spaces with a character of your choice.
Note. The ljust() method creates a new string. It doesn't modify the value of the original string.
But what if our string's already too long for the length we want?
If the original string is longer than the character count you specify in the ljust() method, the method simply returns the original string in its entirety.
Let's see what happens when we tell Python we want our "Hello" string to be just 3 characters long:
>>> text.ljust(3, "*")
Well, "Hello" is five letters, right?
So, Python's just going to give you back your original string "Hello".
Hello
It doesn't cut it down to 3 characters.
And there you have it - the ljust() method in Python, neat and tidy!