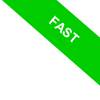
The find() Method for Strings in Python
In this Python course lesson, we'll dive into using the find() method with strings. You'll find it super useful for searching text within string variables.
So, what's the find() method all about? Well, imagine you have a text string like "Hello world". The find() method can help you check if the word "world" is in the text, and pinpoint its position. Pretty neat, right?
Here's a quick rundown of the find() method for string objects.
string.find(substring, from, to)
Breaking down the find() method syntax:
- string: The string object, which is just the text you're working with.
- substring: The string you're trying to locate in the text.
- start: The optional starting position for the search.
By default, it's the first character of the string.
- end: The optional ending position for the search.
By default, it's the last character of the string.
The find() method searches for the substring within the text and returns the position of its first occurrence.
If it doesn't find the substring, it returns "-1".
Quick note. In Python, the first character of a string is at position zero, the second at position one, and so on. Just remember that the index of characters in a string always starts from zero.
Let's see the find() method in action with a practical example.
Create a string called "text".
>>> text = "My father's father"
Now type text.find("father") to search for the string "father" in the text.
>>> text.find("father")
Voilà! The string "father" starts at the fourth character of the text, in index position 3.
Using the find() method in Python, it finds the word "father" within the variable and returns the position of the first letter of the word, which is 3.
3
Heads up. There are two occurrences of the string "father" in the text. The find() method only returns the position of the first occurrence.
Now, let's search for the string "father" in the text starting from a different position.
>>> text.find("father", 5)
The method searches for the word "father" starting from index position 5, which is the sixth character.
In this case, the first occurrence of the string "father" is found at position 12.
The find() method outputs the number 12.
12
Lastly, let's search for the string "father" within a specific range.
Type text.find("father", 5, 14)
>>> text.find("father", 5, 14)
The method searches for the word "father" between positions 5 and 14 of the string.
Unfortunately, the method doesn't find the substring in this case.
So, it outputs the number -1
-1
Remember. When the find() method returns zero (0), it means that it found the substring you're looking for right at the beginning of the text. So, don't mix up 0 with -1.
Looking for a way to find all occurrences of a specific string within a variable?
Let me show you how you can do this using Python's find() method in a friendly and straightforward manner!
First, you'll need to set up a loop to search for the desired string in your text:
- text = "My father's father"
- word_to_search = "father"
- # Find the position of the first occurrence
- position = text.find(word_to_search)
- # Keep looping until no more occurrences are found
- while position != -1:
- print(position)
- position = text.find(word_to_search, position + 1)
So, how does this loop work its magic? It iterates through the text, performing multiple searches. Each search starts from the position of the last occurrence found. With each iteration, the loop dives deeper into the remaining text to find more matches. The search comes to an end when the find() method returns -1, indicating that the string isn't present in the remaining text.
In our example, the loop discovers all instances of the string "father" within the "text" variable and provides a list with the positions of each occurrence.
The search uncovers two matches.
Our script shows the positions 3 and 12, like this:
3
12
So, there you have it! The find() method in Python is a handy and effective tool for searching strings within a text variable. It's a great asset to have in your programming toolbox!