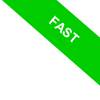
The Split Method for Strings in Python
Are you ready to dive into Python's string split() method? This tutorial will guide you through it.
So, what's the scoop on the split method? It's essentially a tool that enables you to disassemble a string into multiple parts, based on a specific delimiter character. For instance, if you've got a string that reads "Hello world", the split method will split it into two substrings - "Hello" and "world", using a space as the dividing line.
The split() method is an inherent feature of string objects.
string.split(separator,maxsplit)
Here's how the syntax of the split method works:
- The string is what you're applying the method to. It's the chunk of text you want to dissect.
- The separator (enclosed in parentheses) is the delimiter character you choose for slicing up the string. This could also be a sequence of characters. Keep in mind, it's optional.
Quick heads-up: If you don't provide a delimiter character, Python automatically defaults to using whitespace as the separator.
- The maxsplit parameter is the cap on how many times you want the string split. Again, this is optional.
Just to note: If maxsplit is left unspecified, Python will split the string as many times as possible.
Let's make it real with a practical example.
First off, create a string called "text".
>>> text = "Welcome to the wonderful world of Python programming"
Now, by typing text.split(), you'll divide the string into a list of words
>>> text.split()
Here, you didn't denote a separator character in the split method, so Python uses space as the default delimiter to break down the string.
What you'll get is a neat list of words.
['Welcome', 'to', 'the', 'wonderful', 'world', 'of', 'programming', 'in', 'Python']
Let's shake things up with another example.
Set up the string like so:
>>> text = "apple,pear,banana,kiwi"
Now, type text.split(",") to carve up the string using a comma as the separator.
>>> testo.split(",")
This time around, Python separates the string using the comma character ",", stepping away from the space separator.
The endgame? A fresh list of fruits.
['mela', 'pera', 'banana', 'kiwi']
Keep in mind: The separator character will always vanish in the final result. This means if you use a character as a delimiter, it won't appear in your list of results. For example, in the strings "apple" and "pear", the comma that once kept them apart is no more.
The split() method also gives you the option to limit the number of splits by using the maxsplit parameter.
Say you type text.split(",",3)
>>> text.split(",",3)
In this scenario, Python breaks up the string based on the comma character ",", but it only allows for a maximum of three splits.
The result? A list featuring three strings instead of four.
['apple', 'pear', 'banana,kiwi']
The last element "banana,kiwi" remained intact because you limited the number of splits to three.
What's more, the separator can also be a string
For instance, set up the string like this:
>>> text "The book I lent you"
Then type text.split("I")
>>> text.split("lent")
Python then divides the string in two, using "lent" as the separator
The output is a list with two parts
['The book I', 'you']
Notice how the term "lent" isn't included in the list? That's because it was used as the string separator.
Wrapping it all up, the split() method is a powerful tool that provides the capability to fragment a string into multiple sections and independently process each text chunk.