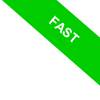
Python's zfill() Method
Have you ever had a situation where you needed to pad a string with zeros on the left until it reaches a certain length? Well, Python's got you covered with its zfill() method. Here's how it works:
string.zfill(length)
What goes in the parentheses? That's where you specify the total number of characters you want in your resulting string, zeros included.
The outcome? You get a string just as long as you asked for, nicely padded with zeros to the left.
But here's a quick heads up. If your string is already longer than the length you've put in the parentheses, zfill() will give you your original string back, without any extra zeros.
Let's make it clearer with an example.
Say, we've got this string assigned to the variable "text".
text = "27"
Then we run zfill(6) on "text", storing the result in another variable "text2".
text2 = text.zfill(6)
Next, we print what's in "text2":
print(text2)
And voila! We end up with a six-character string where the original "text" is padded with zeros on the left:
000027
The zfill() method is perfect for when you want your string to have a fixed length, padding it out with zeros on the left.
It's particularly handy for displaying numbers with a fixed width in outputs.
Remember though, if your original string is longer than the length you specify, zfill() won't tack on any extra zeros. Take for example a 9-character string assigned to the "text" variable:
text = "123456789"
Then we try to run zfill(6) and print the result:
text2 = text.zfill(6)
print(text2)
What do we get? The zfill() method simply returns our original string intact.
123456789
That's Python's zfill() method for you. A useful tool for formatting your strings just the way you want them!