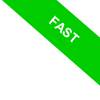
The index() Method for Strings in Python
In Python, the index() method is a handy tool that helps you find the position of the first occurrence of a substring within a larger string. Here's how it works:
string.index(substring, start, end)
The method is applied to the string object and has three parameters:
- Substring: The substring you're looking for within the larger string.
- Start: The starting position (start) in the index from which to kick off the search within the string. If you don't specify this, the search starts from the beginning of the string.
- End: The ending position (end) in the index where the search should wrap up within the string. If you don't specify this, the search ends at the end of the string.
You can use the index() method on any string object in Python, and it'll return the index position of the substring within the larger string.
However, if the substring isn't present within the string, the method will return an error.
Keep in mind that the starting and ending positions for the search are optional parameters. So, if you want to perform a thorough search throughout the string, you can leave these two parameters out.
Let's dive into a practical example.
Try typing in this code:
>>> string = "My father's father"
>>> string.index('father')
In this case, the string.index('father') method searches for the first occurrence of the substring 'father' within the string.
It finds it at index position 3. Indeed, the first "father" substring starts at position 3 in the string's index.
3
Note that there are two "father" substrings in the string, but the index method only returns the position of the first occurrence.
It's essential to remember that, in Python, indices start at 0. This means the first character of the string has an index of 0, the second character has an index of 1, the third character has an index of 2, and so on.
Now, let's try another example. Type in this code:
>>> string = "My father's father"
>>> string.index('father', 5)
Here, the string.index('father', 5) method searches for the first occurrence of the substring 'father' within the string, starting the search at index position 5.
In this case, the first occurrence of the substring "father" is found at position 12.
12
Lastly, try typing in this code:
>>> string = "My father's father"
>>> string.index('father', 5, 15)
The string.index('father', 5, 15) method searches for the first occurrence of the substring 'father' within the string between index positions 5 and 15.
In this case, the search doesn't find the substring "father" and returns an error: ValueError: substring not found.
ValueError: substring not found
Keep in mind that the index() method only returns the position of the first occurrence of the substring within the larger string.
If you want to find all occurrences of the substring, you should use the find() method or a regex expression.
And if you're interested in finding only the position of the last occurrence, give the rindex() method a try.