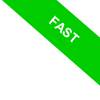
Python's isnumeric() Method
Are you knee-deep in Python and looking for a neat way to find out whether a string is purely numeric? Well, that's where Python's isnumeric() method comes into play. Here's how it works:
>>> string.isnumeric()
All you need to do is call it on your chosen string.
If your string is playing host to numeric characters only, this smart little method will return True. On the other hand, you'll get a False.
Let's go through an example together.
First up, let's create a String type variable. Let's call it "myVar".
>>> myVar = "1234567890"
Now, let's see what happens when we call the isnumeric() method on myVar.
>>> myVar.isnumeric()
Voila! The method returns True. Why? Because myVar is a straight-up numbers-only affair.
True
But let's mix things up a bit.
Now, type in a string with a decimal point.
>>> myVar = "123.45"
And let's give isnumeric() another whirl.
>>> myVar.isnumeric()
This time, isnumeric() returns False. That's because it doesn't see the decimal point as a numeric character.
False
But wait, there's more! The isnumeric() method has a few more tricks up its sleeve. For instance, it can recognize CJK (Chinese, Japanese, and Korean) numbers.
So, if you're juggling texts in multiple languages, this could be a real lifesaver.
More Tools in Your Python Toolbox: isdigit() and isdecimal()
Remember, isnumeric() isn't the only tool you've got for checking if a string has numbers.
The isdigit() and isdecimal() methods are also there, ready to lend a hand.
But remember, each method has its own quirks.
Let's break it down:
- isnumeric() is your go-to if your string might contain non-standard Western numbers, including those nifty CJK (Chinese, Japanese, and Korean) numbers.
- isdigit(), on the other hand, sticks to the script and only recognizes standard Western numbers.
Let's make it crystal clear with some examples.
Example 1
Take the Unicode character "½".
number = "½"
print(number.isnumeric())
print(number.isdecimal())
print(number.isdigit())
isnumeric() sees "½" as a number, but isdecimal() and isdigit() aren't convinced.
True
False
False
Example 2
How about the character "2²" with numbers in superscript?
number = "2²"
print(number.isnumeric())
print(number.isdecimal())
print(number.isdigit())
Here, both isnumeric() and isdecimal() give it the thumbs up, but isdigit() disagrees.
True
False
True
Example 3
Lastly, let's take "六", the Japanese character for the number 5.
number ="六"
print(number.isnumeric())
print(number.isdecimal())
print(number.isdigit())
Again, isnumeric() acknowledges it as a number, but isdecimal() and isdigit() don't.
True
False
False
So there you have it. When it comes to picking the right method, it really boils down to what you need for your specific task.