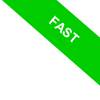
The rfind() Method in Python
Let's delve into the world of Python and one of its string methods: rfind(). This handy method is all about searching for a substring within a string, then giving us the position of its last appearance. Here's how to use it:
string.rfind(substring, [start, [end]])
In this context, "string" refers to the String object where we're hunting for our substring.
There are three parameters in the rfind() method:
- First off, the substring you're tracking down within the string.
- Secondly, 'start' - this is the index from where your search kicks off. It's totally optional. If you leave it out, the search will begin from the string's tail end.
- Thirdly, 'end' - the finish line for the search. Also optional. Leave it out, and the search will wrap up at the string's very first character.
The search is initiated from the string's final position, working its way towards the beginning.
The end goal of the method? To return the index of the last time the substring shows up within the string.
If the rfind() method draws a blank and can't find the substring within the string, it'll return -1.
Here's a heads up: If the rfind() method gives you a 0, it's found the substring right at the start of the string. If it can't find the substring at all, you'll see -1. It's worth keeping these distinctions clear in your mind. After all, in the Python world, a string's first character always has an index of zero.
Now, let's see the rfind() method in action.
First, we'll assign a string to a variable - let's call it "myVar".
myVar = "hello world"
Next, we use the rfind() method to locate the position of the substring "o" in the string, and put the result in a variable named "pos".
pos = myVar.rfind("o")
To finish up, we print the result of our search.
print(pos)
In this instance, our result is 7. Why? Because that's where the substring "o" first pops up in the index.
7
There are actually two instances of the substring "o" in this string - at positions 4 and 7.
The rfind() method only bothers with the last occurrence. It's because the method always searches from right to left within the string, starting from the last character and working its way back to the first.