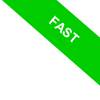
Python's casefold() Method
The casefold() method in Python is a powerful tool for converting all characters in a string to lowercase. This feature is particularly useful for case-insensitive comparisons, allowing you to match strings without the complications of upper and lower case distinctions.
string.casefold()
In this context, string refers to the specific text string you are applying the method to.
One of the key benefits of this method is that it returns a lowercase version of the string while keeping the original string intact.
Casefold's utility shines in various programming scenarios, such as user authentication, text searches, and alphanumeric ordering, where ignoring case differences is crucial.
Let's consider a real-world example of case-insensitive comparison.
Take these two text strings for instance:
str1 = "Python"
str2 = "python"
Although both strings spell "python," the first one starts with an uppercase 'P', setting them apart.
A direct comparison in Python flags them as False due to this difference.
print(str1 == str2)
False
In many situations, such as database searches or web queries, the case difference is irrelevant and should be overlooked.
For a case-blind comparison, apply the casefold() method to both strings:
print(str1.casefold() == str2.casefold())
By converting all characters to lowercase, casefold() aligns the strings, resulting in a positive comparison. Hence, the strings are recognized as identical.
True
Aside from comparisons and searches, casefold is invaluable in string sorting operations.
Distinguishing between lower(), upper(), and casefold()
Similar outcomes can be achieved using the lower() or upper() methods.
str1 = "Python"
str2 = "python"
print(str1.lower() == str2.lower())
The outcome of this comparison is also True
True
Yet, casefold() is often the preferred choice for a couple of reasons:
- It's specifically engineered to remove case sensitivity in strings, making it ideal for more nuanced comparisons.
- Additionally, casefold() supports Unicode characters, making it versatile across different languages and alphabets.
Consider the German word "Straße" as an example:
s1="Straße"
s2="Strasse"
In German, the ß character is always lowercase, and its uppercase equivalent is a double SS.
Using the lower() method for comparison yields a False result because s1 becomes "straße" and s2 "strasse".
print(s1.lower() == s2.lower())
False
Conversely, employing the caseFold() method for the same comparison results in True, as it standardizes both s1 and s2 to "strasse".
print(s1.casefold() == s2.casefold())
True
This illustrates why casefold() is generally the superior choice for case-insensitive string comparisons.