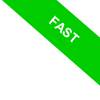
Python's translate() Method
Ever wondered about swapping characters in a string in Python? That's where Python's translate() method comes into play. This method switches out characters in a string, using what we call a translation table. Here's the basic syntax:
string.translate(table)
You can apply this to any String object.
The translation table - the guidebook for what characters get replaced with what - goes inside the round brackets.
This translate() method then works replacing the string's characters with those you've detailed in your translation table.
A quick note: you craft this translation table using the maketrans() method. It outputs a translation table, which you can then pair up with the translate() method.
Let's walk through an example to see this in action.
Imagine you have a string that you assign to the variable "text":
text = 'a b c d e f g h i'
Next, you craft your translation table using the maketrans() method and assign it to the variable "table":
table = str.maketrans('adg', 'xyz')
In this situation, the translation table will replace 'a' with 'x', 'd' with 'y', and 'g' with 'z'.
Now, let's run the translate() method with our translation table to switch out the characters in the string assigned to the "text" object:
result = text.translate(table)
And the final step: print the result to see your new, transformed string:
print(result)
You'll notice that our method has niftily replaced the characters "a", "d", "g" in the string with "x", "y", "z" respectively.
The final outcome? Your string is now:
x b c y e f z h i
And there you have it! Python's translate() method: a simple, effective tool for swapping out characters in a string.