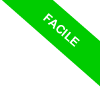
Byte Strings in Python
In Python, byte strings are more than just sequences of characters; they're versatile collections of bytes, capable of representing not just text, but a wide range of data including images, sounds, and more.
Understanding Byte Strings Picture a byte string as a series of compartments, each holding a byte—a tiny packet of information. These bytes can encapsulate a plethora of data types, far beyond mere text, allowing for digital encoding of diverse information forms.
To craft a byte string in Python, prepend a regular string with the b prefix.
For example, b"Ciao" forms a byte string encapsulating the word "Ciao".
message = b"Ciao"
Exploring this string's data type with Python's type() function reveals something interesting:
print(type(message))
Instead of the typical <class 'str'> for text strings, Python identifies it as <class 'byte'>.
<class 'byte'>
Byte Strings vs. Text Strings
Byte strings diverge from standard text strings in their data handling and storage approach. While text strings store familiar characters and symbols, byte strings represent data as numeric sequences, corresponding to bytes. This allows for encoding of non-visible elements and non-textual data.
Their Practical Application Byte strings shine in scenarios like handling binary files, audio, images, or transmitting data over networks in non-text formats. They are akin to a specialized language, interpretable only through the lens of binary coding, contrasting with the everyday language of text strings.
Let's delve into some hands-on examples.
Begin by defining a byte string and assigning it to a variable.
message = b"abc"
Accessing the first character (at index zero) unveils its byte nature:
print(message[0])
Python doesn't show the character "a", but rather its byte representation.
97
This number, 97, corresponds to the "a" character's Unicode code.
Now, observe the byte string's full spectrum and its hexadecimal representation:
- for item in message:
- print(chr(item), item, hex(item))
This loop outputs the characters "a", "b", "c", and their byte equivalents.
a 97 0x61
b 98 0x62
c 99 0x63
These hexadecimal values represent each character's unique byte code, such as x61 for "a", x62 for "b", and so forth.
Decoding Hexadecimal Byte Values
Understanding these values allows for string creation using hexadecimal syntax \xhh, with hh being the byte's Unicode hexadecimal value. This proves invaluable for embedding non-textual data or invisible characters in a string.
For instance, create the same byte string using direct hexadecimal bytes:
newmessage=b"\x61\x62\x63"
Printing this variable displays the familiar string:
print(newmessage)
b'abc'
Similarly, slicing the byte string retains its integrity:
print(newmessage[0:3])
<p> </p>
<p>13 / 12 / 2023</p>b'abc'
Yet, accessing individual characters reveals their underlying numeric codes.
print(newmessage[0])
97
This concise guide demystifies byte strings in Python, highlighting their usage and significance in the realm of programming.