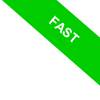
Python's fromhex() Function
In Python, the fromhex() function plays a crucial role in converting hexadecimal strings to byte sequences.
fromhex(string)
The 'string' parameter here refers to the hexadecimal string to be converted.
Depending on which class calls this method, the function returns either a bytes or bytearray object.
To use this function effectively, ensure your hexadecimal string is comprised solely of hexadecimal digits (0-9, a-f, A-F). Including spaces can make it more readable. Note that the string’s length (excluding spaces) should be even, since each pair of digits corresponds to a single byte.
Let's delve into some real-world examples for clarity.
Start by defining a hexadecimal code sequence:
hex_data = "68656c6c6f"
This string's hexadecimal codes correspond to:
- 68: the letter "h"
- 65: the letter "e"
- 6c: the letter "l"
- 6f: the letter "o"
Next, use the fromhex() function to transform these codes into a byte string.
byte_data = bytes.fromhex(hex_data)
Display the byte string:
print(byte_data)
The output is the familiar byte string "hello"
b'hello'
In this example, we've successfully converted a hexadecimal string into a byte string.
Interestingly, the same result can be achieved by spacing out the hexadecimal codes, enhancing readability without affecting the output.
hex_data = "68 65 6c 6c 6f"
byte_data = bytes.fromhex(hex_data)
print(byte_data)
The end result remains unchanged.
b'hello'
Beyond byte strings, the fromhex() function can also be utilized for creating a bytearray object.
hex_data = "68 65 6c 6c 6f"
byte_array_data = bytearray.fromhex(hex_data)
print(byte_array_data)
The outcome here is a bytearray object.
bytearray(b'hello')
In essence, fromhex() is invaluable for converting hexadecimal string representations into bytes, which is essential for tasks like analyzing network packets or binary file content.
It's important to avoid errors, such as an odd-length string or non-hexadecimal characters, as these will lead to a ValueError. Remember, fromhex() is specifically for converting hexadecimal strings to binary data, and not suited for other numeric formats or non-hexadecimal strings.
Conversely, to decipher the hexadecimal representation of bytes or bytearray objects, you can reverse the process using methods like ord() and hex().
For instance, to determine the hexadecimal value of the character "h", you can execute:
print(hex(ord("h")))
This yields:
0x68
Indicating that the hexadecimal code for "h" is 68.