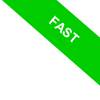
Python's rsplit() Method
Ever wondered how to chop up a string in Python using your own character as the delimiter? That's where the rsplit() method comes into play. Here's how it works:
string.rsplit(sep, maxsplit)
You've got a string, and you call the rsplit() method on it.
The method takes two parameters:
- "sep": this is the character you'll use to break up your string. If you leave it out, it'll default to a space.
- "maxsplit": this is the maximum number of substrings you want to make. It's totally optional - if you skip it, the method will chop away until there's nothing left to chop.
This methos serves up a list of all the pieces, starting from the right and moving towards the left.
Something to keep in mind: rsplit() starts its work from the end of the string, working right-to-left. That's its special trick, setting it apart from the function split(), which works from the beginning of the string, left-to-right.
Let's see rsplit() in action.
Say we've got a string assigned to the variable "text".
text = "Hello, how are you doing today?"
We call rsplit() on text, using a space " " as the chopper.
words = text.rsplit()
Now "words" holds a list of all the words from the sentence.
['Hello,', 'how', 'are', 'you', 'doing', 'today?']
You can tell rsplit() how many chops to make by specifying a number in the second parameter.
For example, type text.rsplit(" ", 2)
words = text.rsplit(" ", 2)
Here, rsplit() serves up three pieces, chopped from right to left.
['Hello, how are you', 'doing', 'today?']
You're not stuck with spaces - you can use any character you like to make your chops.
For instance, let's assign a string to the variable "path".
path = "C:/Users/John/Documents/Report.docx"
Then we'll call rsplit(), using the "/" character as the chopper, and assign the result to "parts".
parts = path.rsplit(sep="/", maxsplit=2)
Print out the content of "parts", and you'll see:
print(parts)
The variable "parts" now holds a list of three pieces, made by chopping the original string twice from the right, using "/" as the chopper.
['C:/Users', 'John', 'Documents/Report.docx']
And just like that, you've got a powerful tool to slice and dice your strings any which way you want!