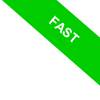
Python's encode() Method
Have you ever needed to convert character strings into an encoded format? Python's got you covered with the handy encode() method!
Here's the breakdown of how it works:
string.encode(encoding, errors)
There are two parameters you'll need to provide within the parentheses:
- "encoding"
This is where you specify the type of encoding you'd like to use. - "errors"
This parameter helps you control how any encoding errors are handled.
Once you run the method, you'll get the encoded version of the string. Pretty neat, right?
Keep in mind that this method is a lifesaver when you need to store strings in a database or a text file. Encoding makes sure your data is saved in a compatible format, which is essential for data storage.
Let me walk you through a real-life example.
Imagine you have a string called "data".
>>> data = "Hello world"
Now, you want to encode the string using the encode() method and specify UTF-8 as the encoding:
>>> data.encode('utf-8')
And there you have it! The encode() method with the 'utf-8' argument encodes the string just like you asked.
In this case, you'll get the "Hello world" string in UTF-8 encoding:
b'Hello world'
But wait, there's more! When you're dealing with data from external sources, you might run into encoding issues that can cause errors during data processing. No worries, though—the encode method has your back! It'll help you handle exceptions and make sure your data is processed correctly.
Let's dive into another example.
Try running this command:
>>> data.encode(encoding="UTF-8", errors="strict")
With the "errors" parameter, you can choose how to handle any encoding errors that might pop up:
- "ignore"
The method will overlook any unencodable characters. - "replace"
The method will swap out unencodable characters with a substitute character. - "strict"
The method will raise an exception if there are any invalid bytes in the string (this is the default setting).
In this case, you'll still get the same result:
b'Hello world'
And there you have it! This nifty little method allows you to tackle errors that might happen during the encoding process. Happy coding!