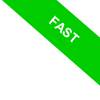
Abstract Classes in Python
In this lesson, we will learn how to use abstract classes in Python.
What is an abstract class? An abstract class is a class that you cannot instantiate directly. It serves as a blueprint for defining the interface of other classes. In other words, an abstract class defines methods (called abstract methods) that must be implemented by derived classes. This ensures that all subclasses adhere to the same common interface.
Abstract classes allow you to define a clear structure for how derived classes should be implemented.
They essentially require subclasses to implement specific methods.
How do you define an abstract class in Python?
In Python, you can create abstract classes using the `abc` (Abstract Base Classes) module. Here's how:
from abc import ABC, abstractmethod
Once you've imported the module, you can define an abstract class and its abstract methods.
class AbstractClass(ABC):
@abstractmethod
def abstract_method(self):
pass
All classes that inherit from this abstract base class must implement the method defined as abstract.
This guarantees that all subclasses will share a common interface.
Practical Example
Imagine you are designing a system to manage different types of animals in a game.
You want to ensure that every animal has basic functionalities like eating and sleeping, but each animal should implement these functionalities differently.
First, you need to define an abstract class and its methods.
For example, define the abstract class 'Animal' which contains two abstract methods: eat() and sleep()
class Animal(ABC):
@abstractmethod
def eat(self):
pass
@abstractmethod
def sleep(self):
pass
Both methods are abstract because they are preceded by @abstractmethod.
Suppose you want to implement two derived classes: `Cat` and `Dog`.
Both classes must implement the `eat` and `sleep` methods.
class Cat(Animal):
def eat(self):
print("The cat eats kibble.")
def sleep(self):
print("The cat sleeps in the basket.")
class Dog(Animal):
def eat(self):
print("The dog eats meat.")
def sleep(self):
print("The dog sleeps in the kennel.")
Now you can create instances of `Cat` and `Dog` and use their methods.
For example, create an instance of the Cat class
cat = Cat()
Then call the eat() method
cat.eat()
The cat eats kibble.
Also, call the sleep() method
cat.sleep()
The cat sleeps in the basket.
Now create an instance of the Dog class.
dog = Dog()
Then call the eat() method
dog.eat()
The dog eats meat.
And the sleep() method
dog.sleep()
The dog sleeps in the kennel.
Both classes have the same methods but different implementations, meaning they share the same interface.
However, you cannot create an instance of `Animal` directly because it is an abstract class.
If you try to instantiate the abstract class directly, it will generate an error
animal = Animal()
TypeError: Can't instantiate abstract class Animal with abstract methods eat, sleep
Adding Concrete Methods
Abstract classes can also contain concrete methods (i.e., methods with an implementation).
For example, you can add a `description` method that provides a generic description of the animal.
class Animal(ABC):
@abstractmethod
def eat(self):
pass
@abstractmethod
def sleep(self):
pass
def description(self):
print("This is an animal.")
In this example, the description() method is not abstract because it is not preceded by @abstractmethod.
Then create two subclasses derived from the abstract class 'Animal'
The 'Cat' subclass
class Cat(Animal):
def eat(self):
print("The cat eats kibble.")
def sleep(self):
print("The cat sleeps in the basket.")
The 'Dog' subclass
class Dog(Animal):
def eat(self):
print("The dog eats meat.")
def sleep(self):
print("The dog sleeps in the kennel.")
Now create an instance of the 'Cat' class
cat = Cat()
Then call the description() method
cat.description()
This is an animal.
The description() method executes even though it is not implemented because it is not an abstract method.
The same happens if you create an instance of the 'Dog' class
dog = Dog()
And then call the description() method, the same method is executed
dog.description()
This is an animal.
In conclusion, abstract classes provide a way to define a common interface for a group of related classes and ensure that these classes implement specific methods.
When used correctly, you can create systems that are easy to understand, extend, and maintain.
I hope this guide was helpful! If you have any questions or want to delve deeper into a topic, let me know!