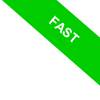
The __repr__ Method in Python Classes
Today, we'll discuss the __repr__ method in Python. This method lets you define how an object is represented when the repr() function is called.
__repr__
It's primarily used for debugging, providing a clear and informative string representation of objects.
When called, it returns a string that represents the object.
The main goal of `__repr__` is to provide a string that could ideally be used to recreate the object. In other words, if you copy and paste the output of `__repr__` into Python code, it should create an identical object.
Let's look at a practical example.
Suppose you have a class `Person`.
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __repr__(self):
return f"Person(name='{self.name}', age={self.age})"
In this class, the __repr__ method is implemented to return a string that shows the class name and the instance attribute values.
Create an instance of the class
student = Person('Alice', 30)
Now access the object's content via the repr() function
print(repr(student))
The output will be a string:
Person(name='Alice', age=30)
As you can see, the output is clear and informative. Now you know that the object is an instance of the `Person` class with the name 'Alice' and the age 30.
Let's look at another more complex example.
Define a `Book` class implementing the __repr__ method.
class Book:
def __init__(self, title, author, pages):
self.title = title
self.author = author
self.pages = pages
def __repr__(self):
return (f"Book(title='{self.title}', author='{self.author}', "
f"pages={self.pages})")
Now create an instance of `Book`
book = Book('The Pleasure of Finding Things Out', 'Richard Feynman', 320)
Finally, access the `__repr__` method via the repr() function.
print(repr(book))
Book(title='The Pleasure of Finding Things Out', author='Richard Feynman', pages=320)
The method displays all the information you asked it to show.
Generally, developers aim to make the output of `__repr__` something that can be copied and pasted into code to create a new object. Therefore, it's very important that the output is clear, useful, and complete, including all necessary information to recreate the object.
As you might have already noticed, this method serves a similar purpose to the __str__ method.
So, it's natural to wonder what the difference between these two methods is. Let's address this question in the next section.
The Difference Between __repr__ and __str__ Methods
These two methods serve to represent an object as a string, but they have slightly different purposes.
- The __repr__ Method
Called by the repr() function, it is mainly used by developers. Ideally, it should be a string that, if passed to eval(), recreates the object. - The __str__ Method
Called by the print() and str() functions, it is mainly intended for end-users. It returns a string that describes the object in a readable way.
So, the main differences between these methods are the functions used to execute them and their intended purposes.
Let's get a better understanding of how these two methods work with a practical example.
Define the `Person` class implementing both methods.
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __repr__(self):
return f"Person(name='{self.name}', age={self.age})"
def __str__(self):
return f"{self.name}, {self.age} years old"
Now create an instance of `Person`.
student = Person('Alice', 30)
When you use the repr() function on the 'student' object, Python calls the __repr__ method
print(repr(student))
Person(name='Alice', age=30)
Conversely, when you use the print() function on the same object, Python calls the __str__ method
print(student)
Alice, 30 years old
The same happens when we use the str() function.
print(str(student))
Alice, 30 years old
In conclusion, the difference between the two methods is primarily their intended purpose.
For the __repr__ method, the string is a useful representation for debugging, whereas for the __str__ method, it is a readable and user-friendly representation intended for end-users.
Fall-Back Mechanism. If a class only implements `__repr__`, `str()` will use `__repr__` as a fallback. The output result is the same. However, it is good programming practice to define both if their purposes differ.
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __repr__(self):
return f"Person(name='{self.name}', age={self.age})"
Create an instance of the class
student = Person('Alice', 30)
Now try to access the object's content via the str() function
print(str(student))
Person(name='Alice', age=30)
In this case, Python automatically calls the __repr__ method because the __str__ method is not defined in the class.
Therefore, you can choose to use only one of the two methods.
However, it's recommended to implement both correctly to enhance the clarity and usability of the code.
I hope the differences are clearer now!