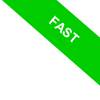
Using Getters and Setters in Python
In programming, getters and setters are crucial for accessing and modifying an object's attribute values. These methods are pivotal for implementing encapsulation—one of the fundamental principles of object-oriented programming. Encapsulation safeguards an object's internal state by exposing only essential data through a controlled interface.
What's their purpose? Even though Python doesn't strictly enforce encapsulation like Java or C++, using getters and setters can obscure the internal representation of an object's data, offering an additional layer of data protection.
Additionally, these methods enable the implementation of validation checks during data assignment, enhancing data integrity.
Let's examine a practical example.
Consider a simple 'Person' class with two attributes:
class Person:
name=""
age=0
Initially, this class lacks getter and setter methods.
When you instantiate this class, you can freely assign values to the object:
student = Person()
student.name="Mary"
student.age= -10
This example demonstrates that without validation, a negative number (-10) can be assigned to the "age" attribute. Python, without built-in checks for such assignments, accepts this input without complaint.
Furthermore, attributes can be directly accessed by simply referencing their names:
print(student.age)
-10
To tackle these issues, we introduce a constructor method ( __init__ ) within the 'Person' class that includes two private attributes, '_age' and '_name', with a validation step to ensure that the age cannot be negative:
class Person:
# constructor method
def __init__(self, name, age):
if (age>0):
self._name = name
self._age = age
else:
raise ValueError("Age cannot be negative.")
This modification necessitates that both the name and age are specified at instantiation, with preliminary validation of the data:
If an attempt is made to set a negative age, Python will now raise an error.
student = Person("Mary", -10)
ValueError: Age cannot be negative.
This enforces the requirement for valid input.
student = Person("Mary", 20)
Direct access to the 'name' and 'age' attributes is now restricted, as they are private ('_name' and '_age').
print(student.age)
AttributeError: 'Person' object has no attribute 'age'. Did you mean: '_age'?
Note that private attributes only offer partial data protection, as they can still be accessed directly by their complete label like '_age' or '_name', without any checks.
student._age=-20
Now, we add a getter method to retrieve the '_age' attribute and a setter method to modify it, incorporating validation into the setter to prevent negative age values:
class Person:
# constructor method
def __init__(self, name, age):
if (age>0):
self._name = name
self._age = age
else:
raise ValueError("Age cannot be negative.")
@property
def age(self):
# age getter
return self._age
@age.setter
def age(self, new_age):
# age setter with validation
if new_age < 0:
raise ValueError("Age cannot be negative.")
self._age = new_age
These methods ensure that attribute manipulation adheres to established rules, thus maintaining the object's data consistency and integrity.
- The getter method accesses and returns the private '_age' attribute.
- The setter method modifies the '_age' attribute, enforcing the validation rule.
The @property decorator allows a method to function as a read-only property, callable without parentheses like an attribute. The @age.setter defines the setter method, integrating validation logic into the assignment process.
Create an instance of the class and interact with it through these methods:
student = Person("Mary", 20)
Access the 'age' attribute using the getter:
print(student.age)
20
Change the 'age' attribute using the setter:
student.age = 25
Attempting to set a negative age will now trigger a Python ValueError:
student.age = -10
ValueError: Age cannot be negative.
In summary, getters and setters in Python provide a sophisticated mechanism for controlling access to an object's data, promoting better data management and security.