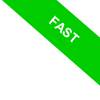
The @property Decorator in Python
In Python, the @property decorator allows you to define class methods that act like attributes.
@property
This means you can access a method as if it were a simple attribute, without needing to use parentheses. This is particularly useful for two main reasons:
- Encapsulation: It helps to hide and protect the state of an instance.
- Access Control: It makes it easier to validate or automatically calculate a value whenever an attribute is accessed or modified.
What’s it for? Using `@property` helps maintain data integrity, especially when the value of an attribute depends on other states or needs to be kept within certain limits. Moreover, it allows you to change the internal implementation without altering how you access or modify the attributes from outside.
Let’s see how to use @property with a practical example.
Create a class `Circle` with an attribute `radius`.
class Circle:
def __init__(self, radius):
self._radius = radius
@property
def area(self):
return 3.14159 * self._radius ** 2
This class calculates the area of the circle each time the radius changes.
As you can see, in the code the attribute is labeled as `_radius` instead of `radius`. This is a convention indicating that this attribute is considered private and should not be directly accessed from outside.
The @property decorator before the `area` method allows you to access the circle's area as if it were an attribute, but it's actually a method that calculates the area each time you request it.
For example, create an instance of the class.
c = Circle(5)
Then access the `area` attribute of the instance.
print(c.area)
78.53975
Python returns the value of the area as if it were an attribute, but in reality, it calculated it in real-time using the `area` attribute.
Thus, even though `area` is technically defined as a method in the Circle class, thanks to the @property decorator, you can access it as if it were an attribute.