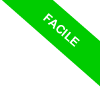
The __init__ Method in Python Classes
In Python, classes are defined with an `__init__` method, commonly referred to as the "constructor." This method is crucial as it initializes newly created objects.
Whenever you create a new instance of a class, Python automatically invokes the `__init__` method, enabling you to establish initial attributes, akin to prepping your ingredients before you start cooking.
The __init__() method, also known as the "initializer," is a function within the object class. This means it's inherited by all classes and can be customized by overriding it.
The first parameter of the __init__ method is always 'self,' which refers to the instance of the object. It's also important to note that this method does not return a value, so including a return statement will cause an error.
A Practical Example
Let's illustrate with a practical example:
Consider a class named `Automobile`.
An automobile possesses several attributes, such as make, model, and color. You can define these attributes in the `__init__` constructor when crafting a new car.
class Automobile:
def __init__(self, make, model, color):
self.make = make
self.model = model
self.color = color
In this example, the `Automobile` class features an `__init__` method that takes three parameters: make, model, and color. These parameters are directly assigned to the object’s attributes—`self.make`, `self.model`, and `self.color`—with `self` referring to the specific object being initialized.
Now, when you instantiate this class, Python expects all necessary information to be provided for the object’s setup. Failing to do so will lead to an error.
For example, you must specify the make, model, and color of the car:
my_car = Automobile("Tesla", "Model S", "black")
Once created, the object is at your disposal.
For instance, accessing its attributes is straightforward:
print(f"My car is a {my_car.make} {my_car.model} in {my_car.color}.")
My car is a Tesla Model S in black.
What if an instance lacks the parameters required by the constructor?
If you attempt to create an object without all necessary values, Python will raise an exception and throw a TypeError, indicating missing arguments.
For instance, omitting the `color` when creating an automobile object will result in an error:
my_car2 = Automobile("Tesla", "Model S")
TypeError: Automobile.__init__() missing 1 required positional argument: 'color'
Overriding Built-in Classes
Overriding the __init__ method allows you to create new data types and structures by extending existing built-in classes.
For instance, you can customize the dict class to create your own specialized dictionary type in Python.
The dict class is one of Python's built-in classes, providing a data structure that maps keys to values.
Suppose you want to create a subclass of dict that adds extra functionality during initialization. For example, you might want the dictionary to automatically include a default key-value pair whenever it's instantiated.
Here’s an example of how you could override the __init__() method in a dict subclass:
class MyDict(dict):
def __init__(self, *args, **kwargs):
# Call the parent class initializer (dict)
super().__init__(*args, **kwargs)
# Add a default key-value pair
self['default_key'] = 'default_value'
This code defines a class called MyDict that inherits methods and properties from Python's dict class while adding some custom behavior to the initializer.
Every time an instance of the MyDict class is created, the dictionary automatically includes the key "default_key" with the value "default_value".
Note that to override __init__(), the base class initializer dict is invoked using super().__init__(*args, **kwargs). This ensures the dictionary is properly initialized, even if additional arguments are passed.
Create an instance of the class:
my_dict = MyDict(a=1, b=2)
Now, display the contents of the object:
print(my_dict)
{'a': 1, 'b': 2, 'default_key': 'default_value'}
In addition to the values passed as arguments, the dictionary also includes the default key-value pair that was automatically added during initialization.
This is a simple example of how you can override the __init__() method in a built-in Python class to extend or alter its standard behavior.
In summary, the `__init__` method is indispensable for initializing objects with their essential attributes, ensuring they are fully functional from the outset. Every time an object is created from this class, `__init__` is called automatically, setting the stage for its use in Python.