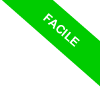
The __prepare__() Method in Python
The `__prepare__` method in Python is invoked before a class is actually created.
What’s its purpose? Its primary function is to return a mapping (an object similar to a dictionary) that Python will use to collect the class attributes. While it may seem complex at first glance, once you get into it, you’ll find it quite valuable if you’re working with metaclasses. It’s one of those features that often flies under the radar.
Normally, a class in Python is created using a metaclass called `type`.
However, if you want to have control over how classes are constructed, you can define your own metaclass. Among the methods in a metaclass is the `__prepare__` method.
Why does it matter?
By default, Python uses a basic dictionary to collect class attributes. But with `__prepare__`, you can return a different mapping—whether it's a specialized data structure or an ordered dictionary.
In essence, it allows you to influence the class creation process at a very low level, giving you the ability to customize how Python handles this process.
For example, this can be particularly useful if you need to control the order in which class attributes are defined, or if you want to perform specific actions during the class creation phase.
A Practical Example
Let’s walk through a concrete example to illustrate this concept.
Imagine you want to create a metaclass that tracks the exact order in which the attributes of a class are defined.
You can define a metaclass called 'MyMeta'.
from collections import OrderedDict
class MyMeta(type):
@classmethod
def __prepare__(metacls, name, bases):
# Returns an OrderedDict to preserve attribute order
return OrderedDict()
def __new__(metacls, name, bases, classdict):
# Creates the class as usual
cls = super().__new__(metacls, name, bases, dict(classdict))
# Prints the attribute order
print(f"Order of attributes for the class {name}:")
for key in classdict:
print(f" {key}")
return cls
This metaclass uses an OrderedDict to ensure that the insertion order of attributes is preserved.
Now, let’s define a class that uses 'MyMeta' as its metaclass:
class MyClass(metaclass=MyMeta):
x = 10
y = 20
z = 30
When the `MyClass` class is created, Python calls the `__prepare__()` method, which returns an `OrderedDict` instead of the default `dict`.
Python then uses this `OrderedDict` to collect all the class attributes (`x`, `y`, `z`).
Finally, it prints the exact order of the attributes.
Order of attributes for the class MyClass:
__module__
__qualname__
x
y
z
In this example, the `__prepare__` method was responsible for controlling how Python gathered the attributes during the class definition process.