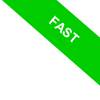
The Constructor Method in Python Classes
The constructor method, automatically executed when a new class instance is created, primarily initializes the instance's attributes. In Python, this method is called `__init__`. Here’s how it's typically written:
class ClassName:
def __init__(self, parameter1, parameter2):
self.attribute1 = parameter1
self.attribute2 = parameter2
The parameters within the parentheses after __init__ are as follows:
- `self` refers to the current instance of the class, enabling access to its attributes and methods.
- the list of parameters passed to the constructor.
The __init__ method is vital as it sets up the instance's attributes right at creation and can also perform additional initialization tasks like data validation, calculations, or establishing default values.
The constructor method is automatically triggered when an object is created and shouldn't be invoked manually. Keep in mind, it runs just once per object at the time of creation. To maintain data consistency and integrity, it’s advisable to include similar checks in getter methods or other methods that alter the object's attributes post-initialization.
Example
Consider the creation of a class `Automobile` that requires attributes such as make, model, and year of manufacture:
Here's a typical constructor:
class Automobile:
def __init__(self, make, model, year):
self.make = make
self.model = model
self.year = year
This constructor explicitly requires that each new instance specify values for make, model, and year.
my_car = Automobile("Tesla", "Model S", 2022)
Omitting any of these attributes when creating an instance will lead Python to halt the process and raise an error.
my_car2 = Automobile()
error
Once instantiated, you can freely access each attribute of the object.
print(my_car.make)
TypeError: Automobile.__init__() missing 3 required positional arguments: 'make', 'model', and 'year'
The constructor method goes beyond simple attribute initialization to offer numerous other benefits.
Default Values
You can assign default values to the constructor’s attributes, which will be used unless explicitly overridden:
class Automobile:
def __init__(self, make, model, year=2024):
self.make = make
self.model = model
self.year = year
If a year isn't specified, the instance is still created with the default year set to 2024.
my_car = Automobile("Tesla", "Model S")
Pulling up the year attribute now, Python will show it as 2024.
print(my_car.year)
2024
Data Validation
The constructor also facilitates data validation, ensuring that inputs meet predefined criteria.
For instance, this class includes a year validation check:
class Automobile:
def __init__(self, make, model, year):
if year < 1990 or year > 2024:
raise ValueError("Year must be between 1990 and 2023")
self.make = make
self.model = model
self.year = year
If the year is outside the acceptable range, Python will not create the instance and will raise an exception.
my_car = Automobile("Tesla", "Model S", 2025)
ValueError: Year must be between 1990 and 2023
This validation ensures the correctness of the data entered.
Performing Calculations During Initialization
The constructor can also execute calculations or invoke other class methods to enhance functionality.
Consider a class 'Rectangle' that computes its area during initialization:
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
self.area = self.calculate_area() # Calculating area in the constructor
def calculate_area(self):
return self.width * self.height
This ensures the area is immediately available as an attribute right after creation.
my_rectangle = Rectangle(4, 5)
Now, check the computed area:
print(my_rectangle.area)
20
This constructor approach effectively reduces the need for additional parameters, simplifying instance creation and enhancing immediate functionality.
In the final example, the area calculation is handled by a dedicated method to keep the constructor simple and focused. This is a best practice for maintaining organized and reusable code. Yet, performing the calculation directly within the constructor is also a viable option, yielding the same result.
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
self.area = self.width * self.height # Calculating area in the constructor
In conclusion, the constructor method is indispensable for crafting and managing Python objects, streamlining programming and ensuring structural clarity.