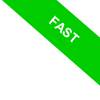
Python's isinstance() Function
The isinstance() function in Python is a powerful tool for checking whether an object belongs to a specific class or type, or falls within a tuple of specified classes or types. Here's how you can use it:
isinstance(object, classinfo)
This function requires two parameters:
- `object` refers to the object you're examining.
- `classinfo` can be a class, a data type, or a tuple containing multiple classes and types you want to compare the object to. If `classinfo` is a tuple, the function returns true if the object matches any class or type listed.
When the object matches the class, subclass, type, or any element within `classinfo` if it's a tuple, isinstance() returns `True`. Otherwise, it returns `False`.
The isinstance() function is particularly handy in object-oriented programming (OOP), enabling quick verification of an object's class or type. It's also great for ensuring an object matches the expected data type.
Here's a closer look at practical uses of isinstance().
For instance, to confirm if a variable `num` holds an integer, you can use:
num=10
print(isinstance(num, int))
True
This approach proves invaluable when crafting functions that handle various input types and need to behave differently based on the input type.
Beyond type checking, isinstance() is excellent for validating data structures.
To verify whether `myList` is indeed a list, for example:
myList=[1,2,3,4,5]
print(isinstance(myList, list))
True
At its core, the isinstance() function is about verifying an object's class membership.
Imagine you have a class named MiaClasse:
class MiaClasse:
pass
After creating an instance of MiaClasse:
mio_oggetto = MiaClasse()
Check its instance like so:
print(isinstance(mio_oggetto, MiaClasse))
The function confirms 'True', affirming the object's class membership.
True
As a cornerstone of Python programming, especially when dealing with complex data structures or polymorphism, `isinstance()` is indispensable. Yet, it's wise to use it