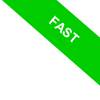
The @setter Decorator in Python
The @setter decorator is a powerful feature in Python that facilitates the creation of an interface to a private attribute within a class.
@setter
It is commonly paired with the @property decorator, which transforms a method into a "getter" attribute, allowing class attributes to be accessed seamlessly as properties.
This configuration ensures that any attempt to initialize or modify the attribute's value triggers an interface that checks and validates the data.
While this topic might initially appear complex, it is incredibly useful for managing how attributes are modified within a class. It allows developers to alter function behaviors without directly changing the underlying code.
Consider a practical example:
Let's create a `Circle` class to model a circle in Python, ensuring that the radius cannot be negative or zero. We'll manage this by utilizing both @property and @setter.
class Circle:
def __init__(self, radius):
self.radius = radius # Set the radius using the setter
@property
def radius(self):
return self._radius
@radius.setter
def radius(self, value):
if value <= 0:
raise ValueError("Radius must be greater than zero.")
self._radius = value
In this code, the constructor `__init__` sets the circle’s radius via the @radius.setter method, which validates the value before storing it in the private attribute `_radius`.
It's important to note that using _radius instead of radius inside the Circle class is a typical Python convention aimed at encapsulation. It serves to conceal the internal workings of the class while exposing only the necessary parts for external use.
If a zero or negative value is provided, an exception is triggered.
For instance, assigning a radius of -5 will cause the constructor to pass this value to the 'radius' setter method, which detects the negative value and throws an exception.
circle = Circle(-5)
ValueError: Radius must be greater than zero.
The combined use of `@property` and `@setter` ensures that the circle’s radius remains positive, safeguarding the integrity of the model.
This methodology affords several advantages:
- Encapsulation: It shields the class properties from improper assignments.
- Data Validation: It allows for the establishment of criteria for data validation when an attribute is changed.
- Interface Simplicity: It keeps the external interface of the class clean and straightforward, as attributes can be accessed directly.
These tools, when skillfully applied, not only enhance the safety of the code but also improve its readability and maintainability.
Mastering their application is key to leveraging their full potential effectively.
In Python, the @setter decorator must be used in conjunction with the @property decorator, as it cannot function independently.
- The @property decorator defines a getter method that behaves like a standard attribute of the class, accessible without parentheses.
- The @setter decorator defines the corresponding setter method for a getter established by @property. This method allows the attribute's value to be modified, incorporating necessary checks or validations.
Why the @setter cannot be used on its own
The @setter is technically part of the infrastructure that the @property decorator establishes. When you define an @setter, it is effectively a secondary definition for the method under the same name as the @property, but decorated with @property_name.setter. Attempting to use @setter without first defining the property with @property would result in Python being unable to recognize to which property the setter refers, leading to a syntax error.