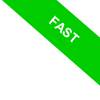
Object Class in Python
In Python, the `object` class is the cornerstone of all classes and sits at the top of the class hierarchy.
This means that every class created is inherently a subclass of `object`, and consequently, every object is an instance of `object`. Regardless of the object's type, they are all instances of `object`.
For example, create a list:
myList = [1, 2, 3]
This list is an object and is an instance of the `object` class.
print(isinstance(myList, object))
True
Even the `type` metaclass, which creates classes, is itself an instance of the base `object` class.
However, `object` has no superclasses, so it stands alone at the top of the hierarchy.
print(isinstance(type, object))
True
And naturally, the base `object` class is also an instance of itself.
print(isinstance(object, object))
True
Every object is an instance of `object`, including simple data types.
For example, assign a value to the variable n:
n = 10
The variable n is an instance of the `object` class.
print(isinstance(n, object))
True
The same principle applies when you create a custom class.
class Foo:
pass
By accessing the __bases__ attribute of the class, you can confirm that it is derived from the `object` class.
print(Foo.__bases__)
(<class 'object'>,)
In conclusion, all classes implicitly inherit from `object`.
This means that all classes in Python, directly or indirectly, are subclasses of `object`, and therefore all objects are instances of `object`.
Some objects are "types" or "classes" if they are also subclasses of the `type` class,