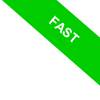
The __enter__() Method in Python
The `__enter__()` method is an essential part of Python's context management protocol, which is often used with the `with` statement.
When dealing with resources that require careful handling, such as files, database connections, or network resources, the context management protocol allows you to automatically manage the acquisition and release of these resources.
Before we delve into the `__enter__()` method, it's important to understand the context in which it's used.
Suppose you need to open a file, read its contents, and then close it. You might write the code like this:
file = open('example.txt', 'r')
data = file.read()
file.close()
In this scenario, if an error occurs while reading the file (for instance, if an exception is raised), the file might not close properly, leaving the resource open.
This is where the `with` statement comes in, ensuring that resources are properly released:
with open('example.txt', 'r') as file:
data = file.read()
# The file is automatically closed when the with block is exited
But how exactly does this work? This is where the `__enter__()` method comes into play.
The `__enter__()` method is part of the class of an object that supports the context management protocol.
When you use the `with` statement, Python automatically calls the object's `__enter__()` method at the start of the block.
Let's look at a practical example by creating a simple class that uses both the `__enter__()` and `__exit__()` methods, with the latter being called at the end of the `with` block to release resources.
class ResourceManager:
def __enter__(self):
print("Entering the context: resource acquired")
return self
def __exit__(self, exc_type, exc_value, traceback):
print("Exiting the context: resource released")
def operation(self):
print("Performing an operation on the resource")
# Using the class with the with block
with ResourceManager() as resource:
resource.operation()
When we enter the `with` block, the `__enter__()` method is called automatically. In this example, it prints a message and returns `self`, allowing us to use the object within the `with` block.
During the `with` block, we can perform operations on the resource.
At the end of the `with` block, regardless of whether an exception was raised, the `__exit__()` method is invoked to release the resource. In this case, it prints another message.
When you run this code, you’ll see the following output:
Entering the context: resource acquired
Performing an operation on the resource
Exiting the context: resource released
In conclusion, the `__enter__()` method is a powerful and invaluable tool for safely managing resources in Python.
By using the context management protocol, you can ensure that resources are released properly, making your code more robust and less prone to errors.