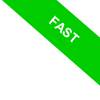
Metaclasses in Python
In Python, a metaclass is a class that defines other classes.
What does a metaclass do? A metaclass controls the creation and behavior of classes, just like classes control the creation and behavior of their instances. Essentially, it allows you to customize how classes are built and instantiated.
When you define a class in Python, the interpreter creates an object of that class, which is usually an instance of the default metaclass `type`.
For example, let's create a class called 'MyClass'.
class MyClass:
pass
Under the hood, Python creates an object using the 'type' metaclass.
MyClass = type('MyClass', (), {})
The call to `type` creates a new class named 'MyClass'.
The new class has no parent classes because the tuple `()` is empty, and it has no attributes since the dictionary `{}` is also empty.
So, 'type' is Python's default metaclass.
However, you can create your own metaclass to customize the class creation process. In fact, you can define custom metaclasses that inherit from `type`.
Defining a Custom Metaclass
To create a custom metaclass, you need to build a class that directly inherits from 'type'.
For example, let's create a metaclass named 'MyMeta'.
class MyMeta(type):
def __new__(cls, name, bases, dct):
print(f'Creating class {name}')
return super().__new__(cls, name, bases, dct)
This custom metaclass inherits from `type` and overrides the `__new__` method to introduce custom behavior during class creation.
The `__new__` method is called before `__init__` and is responsible for returning a new instance of the class.
To use 'MyMeta' as a metaclass when defining a new class, specify it using the metaclass attribute in parentheses.
For example, let's create a new class called 'MyClass'.
class MyClass(metaclass=MyMeta):
pass
Here, 'MyClass' uses `MyMeta` as its metaclass.
This means that when the class is created, the `__new__` method of `MyMeta` is automatically called, printing this message to the screen.
Creating class MyClass
In this simple example, the metaclass's `__new__` method just prints a message. However, you can have it perform any other actions.
A Practical Example
Now, let's explore a practical use case for a custom metaclass.
We’ll define a metaclass to ensure that any new classes using it include a specific attribute.
class MyMeta(type):
def __new__(cls, name, bases, dct):
if 'year' not in dct:
raise TypeError(f"{name} is missing the 'year' attribute")
print(f'Creating class {name}')
return super().__new__(cls, name, bases, dct)
This metaclass checks if the new class defines an attribute called 'year'.
If it's not present, the metaclass prevents the class from being created.
For instance, let's create a new class that uses 'MyMeta' as its metaclass.
class MyClass1(metaclass=MyMeta):
year = 2020
When this class is created, Python invokes the `__new__` method of the metaclass to perform the check.
In this case, the new class includes the 'year' attribute, so the class is created successfully.
Creating class MyClass1
Now, let's attempt to create another class that uses 'MyMeta' but lacks the required attribute.
class MyClass2(metaclass=MyMeta):
day = 27
When you try to create this class without the required 'year' attribute, the 'MyMeta' metaclass raises an error and blocks the class creation.
TypeError: MyClass2 is missing the 'year' attribute
This allows you to control how new classes are created, initialized, and configured.
When Should You Use Metaclasses?
Metaclasses are a powerful and advanced feature in Python, although they are not widely used.
They are best suited for situations where you need to customize class creation.
For example, you can use them to ensure new classes meet certain criteria, contain specific attributes or methods, or automatically apply decorators to all methods in a class.
However, remember that metaclasses can introduce complexity to your code, so use them sparingly and only when absolutely necessary.