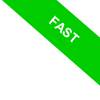
The __delete__() Method in Python
In this lesson, we'll explore the `__delete__()` method used in Python descriptors.
What is `__delete__()`? Think of this method as a "guardian" for an attribute within an object. When you want to delete an attribute, `__delete__()` lets you do more than just remove it—you can use it to perform checks or take specific actions before deletion. However, to use this method, the attribute must be managed through a descriptor class.
Before diving into `__delete__()`, let's first understand what descriptors are.
Descriptors are objects that control access to other attributes in a class. They are classes that implement at least one of these methods:
- `__get__()`: Called when reading the attribute.
- `__set__()`: Called when assigning a value to the attribute.
- `__delete__()`: Called when deleting the attribute.
So, `__delete__()` is a special method that allows you to define what happens when an attribute is deleted.
__delete__(self, instance)
Where:
- `self` refers to the descriptor instance.
- `instance` is the instance of the class containing the attribute.
Why use it? The `__delete__()` method acts as a "final switch" for attributes managed by a descriptor. It lets you control what should happen when an attribute is deleted, giving you full control over the attribute's lifecycle and helping make your code more secure and predictable.
Let’s see how it works with an example!
A Practical Example
Suppose you have a class representing a user, with a `username` attribute.
In this case, it might be useful to ensure that the username cannot be deleted once it’s set. You can achieve this by using the `__delete__()` method.
First, let's create a descriptor class called 'NonDeletable'.
class NonDeletable:
def __init__(self, value):
self._value = value
def __get__(self, instance, owner):
return self._value
def __set__(self, instance, value):
self._value = value
def __delete__(self, instance):
print("Error: You cannot delete this attribute!")
This class implements three methods in addition to its constructor:
- The methods `__get__()` and `__set__()` don't do anything extra, which means you can still read and modify the attribute.
- The `__delete__()` method, instead of deleting the attribute, prints an error message.
Next, let's create a 'User' class and define the 'username' attribute using the 'NonDeletable' descriptor class.
class User:
username = NonDeletable("user1")
def __init__(self, password):
self.password = password
Notice that the 'User' class has two attributes: 'username' and 'password'. However, only 'username' is managed by the descriptor.
Now, create an instance of the 'User' class:
user = User('1234567')
Here, you provide the password '1234567' for the user.
The username 'user1' is set directly in the code for the 'username' attribute.
When you access the 'username' attribute, the assigned value is displayed:
print(f"Username: {user.username}")
Username: user1
Now, try to delete the 'username' attribute using the del statement:
del user.username
When you attempt to delete the attribute, Python calls the `__delete__()` method of the descriptor, which prints an error message and prevents the deletion.
Error: You cannot delete this attribute!
So, even after calling `del user.username`, the `username` attribute remains unchanged.
Running `print(f"Username: {user.username}")` will still show the original username:
print(f"Username: {user.username}")
Username: user1
In this example, `__delete__()` is used to prevent an important attribute (in this case, `username`) from being deleted.
Why Use `__delete__()`?
The `__delete__()` method provides extra control over what happens when an attribute is deleted.
It's particularly useful when you need deletion to be handled in a controlled manner, such as when managing resources (network connections, open files) or sensitive data, where a simple removal might not suffice.
It can also safeguard critical data within a class, ensuring it can't be accidentally removed.
As always, simplicity is key: use `__delete__()` only when it genuinely enhances your class's behavior.