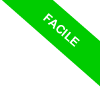
The hasattr() Function in Python
The hasattr() function in Python serves as a handy tool for checking whether an object includes a particular attribute.
hasattr(object, name)
This utility requires two parameters: the object under inspection, and a string that specifies the attribute's name you're interested in verifying.
- object: the target object for the verification.
- name: the attribute's name you wish to check, provided as a string.
If the attribute exists on the object, hasattr() will return True; if not, it returns False.
Utilizing the hasattr() function proves especially beneficial when you're navigating through objects with an unknown structure, like dynamic objects, or when probing for attributes that may not consistently be present due to variations in implementation or initial setup. It also plays a crucial role in preventing your code from encountering an "AttributeError" when it attempts to access an attribute that doesn't exist.
Consider, for example, a "Car" class that includes a "brand" attribute.
With hasattr(), you can easily check whether an instance of this class carries the "brand" attribute.
class Car:
def __init__(self, brand):
self.brand = brand
Create a "my_car" object from the "Car" class.
my_car = Car("Fiat")
Next, determine if the "my_car" object possesses the "brand" attribute by using the hasattr() function.
print(hasattr(my_car, "brand"))
This returns True, indicating that the class indeed has the specified attribute.
True
Now, let's check whether the "my_car" object has a "model" attribute, which hasn't been defined in the class.
print(hasattr(my_car, "model"))
Since the attribute is not found, the function returns False.
False
This approach effectively avoids the risk of an AttributeError by ensuring you only access attributes that exist.