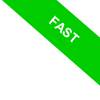
Callable Properties in Python Classes
In Python, the concept of a callable property can be implemented using the `@property` decorator.
@property
This feature allows methods to be treated like non-callable attributes.
Essentially, you can access the results of a method as if it were a static attribute.
Let's look at a concrete example.
class Car:
def __init__(self, make, model):
self._make = make
self._model = model
@property
def description(self):
return f"{self._make} {self._model}"
In this example, `description` is initially set up as a method. However, by using the `@property` decorator, you can access it just like a non-callable property.
For instance, consider creating an instance of the class.
car = Car("Tesla", "Model S")
You can then access the method without the need for parentheses, treating it as a property.
print(car.description)
Tesla Model S
This method of access makes it appear as though `car.description` is a simple property, but it actually invokes car.description() as a method.
This functionality demonstrates Python's flexibility in merging the capabilities of methods and properties into a single, cohesive framework.