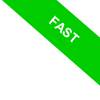
Modifying Class Attributes in Python
Understanding how to manage class attributes in Python is a cornerstone of object-oriented programming. In this guide, I'll walk you through the methods to effectively modify and manage these attributes.
What is a class attribute? A class attribute is a variable that all instances of a class share. It is defined within the class itself but outside any methods. These attributes are perfect for constants or properties common to all instances.
Here's a practical example of a class attribute:
class Car:
doors = 4 # Class attribute
When you instantiate the 'Car' class, all created objects inherit the 'doors' class attribute.
myCar1 = Car()
myCar2 = Car()
For example, querying the 'doors' attribute from the myCar2 instance will return 4.
print(myCar2.doors)
4
Now, let’s explore how to change the class attribute. You can modify it directly or use the setattr() function.
The Direct Method
You can directly alter a class attribute using the class’s name.
For example, to change the 'doors' class attribute in the 'Car' class, simply write:
Car.doors = 5
Python then updates the class with the new value.
print(Car.doors)
5
This change will affect all instances, including those that have not overridden the attribute.
For example, if you check the 'doors' attribute on the myCar2 instance now, it will show 5.
print(myCar2.doors)
5
Avoid modifying the class attribute from an instance. Doing so won't trigger an error, but it will create an instance attribute for that object, leaving the class attribute unchanged. This oversight can lead to subtle bugs. For instance, let's try modifying the 'doors' attribute from the myCar2 instance:
class Car:
doors = 4 # Class attribute
myCar1 = Car()
myCar2 = Car()
myCar2.doors=5 # Instance attribute
Assigning myCar2.doors = 5 creates a new 'doors' attribute just for the myCar2 instance, obscuring the original class attribute for that instance. As a result, if you call the class attribute, its value remains unchanged at 4.
print(Car.doors)
4
Other instances like myCar1 are unaffected by this change and continue displaying the original class attribute value of 4.
print(myCar1.doors)
4
If you call the 'doors' attribute from the myCar2 instance, Python reads the instance-specific attribute and returns 5.
print(myCar2.doors)
5
The setattr() Method
The setattr() function enables you to dynamically modify class attributes.
This method is particularly useful when the attribute name is unknown ahead of time and is determined during script execution.
For example, to change the 'doors' class attribute of the 'Car' class dynamically, you could use:
setattr(Car, 'doors', 6)
The modification is applied directly to the class.
print(Car.doors)
6
All instances automatically inherit the new attribute value.
For instance, if you display the 'doors' attribute from the myCar2 instance now, Python will return 6.
print(myCar2.doors)
6
Adding New Class Attributes
In Python, it is possible to add attributes to a class even after its initialization.
Suppose you have already defined a 'Car' class and created some instances.
class Car:
doors = 4 # Class attribute
myCar1 = Car()
myCar2 = Car()
You can introduce a new class attribute called 'wheels' by simply assigning it.
Car.wheels = 4
Alternatively, you can use the setattr() method to add the attribute:
setattr(Car, 'wheels', 6)
In both scenarios, Python adds the new 'wheels' attribute to the 'Car' class.
print(Car.wheels)
4
This new attribute is automatically inherited by all class instances.
print(myCar2.wheels)
4
Remember, modifying a class attribute impacts all instances unless they have instance attributes with the same name. It’s crucial to grasp this to prevent unintended side effects.
Keeping a clear distinction between class and instance attributes is essential to avoid confusion and potential bugs. Documenting any attribute changes is crucial for helping other developers understand the modifications made to the code.
The Class Attributes Dictionary
Each Python class features a dictionary, __dict__, which enumerates all its related attributes.
This class attributes dictionary is distinct from the __dict__ dictionary of each instance.
Instance dictionaries hold attributes unique to each instance and function independently from the class’s dictionary.
If you have the Car class:
class Car:
doors = 4 # Class attribute
You can review the dictionary by simply typing Car.__dict__.
Among various attributes, you'll find the class attribute 'doors'.
print(Car.__dict__)
{'__module__': '__main__', 'doors': 4, '__dict__':
Unlike an instance's dictionary, the __dict__ of a class in Python is immutable.
This is because the class’s `__dict__` is not a simple dictionary, but a `mappingproxy` object, providing a read-only view of the underlying attributes dictionary.
Attempting to modify the class attribute through the class’s `__dict__` results in an error.
Car.__dict__['doors'] = 5
TypeError: 'mappingproxy' object does not support item assignment
This raises a `TypeError` because you are trying to substitute a `mappingproxy` with a regular dictionary, which is not permitted.
Why does Python use a mappingproxy? The use of a `mappingproxy` was essential with the advent of Python 3 to preserve the integrity of class attributes, safeguarding them from unintended alterations that could affect all class instances. This measure helps prevent hard-to-detect bugs and ensures the code remains secure and maintainable. Previously, in Python 2, the class attribute dictionary was a regular, modifiable dictionary.
To sum up, while you can modify class attributes, it must be done using methods that respect Python’s guidelines, like direct modification (e.g., car.doors=5) or the built-in setattr() method, rather than through the class’s dictionary.
These methods are endorsed because they offer more safety, control, and maintenance ease.
Armed with this knowledge, you are now well-equipped to handle, modify, and add class attributes in Python securely and effectively.