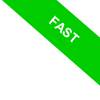
The __exit__() Method in Python
Let's delve into the special `__exit__()` method in Python, a key component of context management that's primarily used with the `with` statement.
What is the `__exit__()` method? In Python, the `__exit__()` method is part of a class that implements the context management protocol. This protocol allows you to manage resources like files or database connections safely and efficiently, ensuring they are properly released after use, even if an error occurs.
When you use a `with` statement, Python looks for the `__enter__()` method in the class, which runs at the start of the `with` block, and the `__exit__()` method, which runs at the end of the block, regardless of whether an exception is raised.
Here’s a general overview to help you understand how it works:
class MyContextManager:
def __enter__(self):
# This code runs at the start of the `with` block
print("Starting context")
return self
def __exit__(self, exc_type, exc_value, traceback):
# This code runs at the end of the `with` block
# exc_type, exc_value, and traceback relate to any exceptions raised
if exc_type is not None:
print(f"An exception occurred: {exc_type}, {exc_value}")
print("Ending context")
return False # Return False to propagate the exception (if any)
Now, let's look at a practical example to see how the `__exit__()` method works in action.
Imagine we have a simple context manager that handles opening and closing a file:
class FileHandler:
def __init__(self, filename, mode):
self.filename = filename
self.mode = mode
self.file = None
def __enter__(self):
self.file = open(self.filename, self.mode)
return self.file
def __exit__(self, exc_type, exc_value, traceback):
if self.file:
self.file.close()
if exc_type is not None:
print(f"Error: {exc_value}")
return True # Return True to suppress the exception
# Using the context
with FileHandler("example.txt", "w") as f:
f.write("Writing something to the file.")
# If an exception is raised here, __exit__() will handle it
# raise ValueError("Test error")
print("File handled and closed properly.")
In this example:
- The `__enter__()` method opens the file and returns it, making it accessible within the `with` block.
- The `__exit__()` method ensures the file is closed, regardless of whether an exception occurs.
- If an exception is raised within the `with` block, the `__exit__()` method still runs and can choose whether to propagate or suppress the exception.
Handling Exceptions. In the `__exit__()` method, the `exc_type`, `exc_value`, and `traceback` parameters provide details about any exception that might have been raised. If `exc_type` is `None`, it means no exceptions occurred. Returning `True` from the `__exit__()` method suppresses the exception, allowing the program to continue normally. Returning `False` allows the exception to propagate.
In summary, the `__exit__()` method is a powerful tool for ensuring that resources are managed properly.
When combined with the `__enter__()` method, it helps you write clean, reliable code that prevents resource leaks and ensures that critical operations are always completed.
Remember, no matter what happens inside the `with` block, Python will always execute the