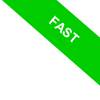
The setattr() Function in Python
The setattr() function is a built-in Python utility that dynamically assigns values to an object's attributes.
setattr(object, name, value)
This function accepts three parameters:
- object: The target object for the attribute assignment or modification.
- name: The name of the attribute as a string.
- value: The value to be assigned to the attribute.
Using setattr(), the value `value` is assigned to the attribute `name` of the object `obj`.
If the attribute doesn't already exist, setattr() will create it and assign the given value `value`.
This function shines in metaprogramming, where attribute names or their values might not be known until runtime, allowing for dynamic adjustments to an object's properties.
It is particularly handy for either modifying or adding attributes to an object.
Modifying an Object's Attribute
Imagine you have a `Car` class with a `color` attribute:
class Car:
def __init__(self, color):
self.color = color
After creating an instance of the Car class,
my_car = Car("red")
you can change its color with setattr().
setattr(my_car, 'color', 'blue')
Checking the attribute afterwards will show the updated color:
print(my_car.color)
blue
This replaces the previous 'color' attribute with the new value in the object my_car.
Creating a New Attribute
When an attribute doesn't exist, the setattr() function can create it on the fly.
Using the same Car class:
class Car:
def __init__(self, color):
self.color = color
and creating an instance:
my_car = Car("red")
you can add a 'doors' attribute using setattr():
setattr(my_car, 'doors', 4)
Now querying this new attribute will return the value you assigned:
print(my_car.doors)
4
Dynamic Attribute Management
The real power of setattr() is seen when dealing with attributes whose names or values are determined at runtime.
In the example below, attribute names and values are stored in variables.
class Car:
def __init__(self, color):
self.color = color
my_car = Car("red")
attribute_name = 'model'
car_model = 'Corolla'
setattr(my_car, attribute_name, car_model)
With setattr(), the script references the variable names instead of directly using attribute names.
This illustrates how dynamic attribute management can simplify handling properties that change at runtime.
Considerations for Dynamic Attribute Management. While extremely useful, dynamic attribute management can make your code less predictable and more complex, particularly when attribute names are generated on the fly. Always validate attribute names and values before applying them with setattr(). Additionally, thorough documentation in your code will aid other developers in understanding your approach more clearly.
Despite the power of the setattr() function, consider using dedicated setter methods for changing object states when practical. These methods offer clearer, more maintainable code and can provide extra safeguards through additional checks and validations.