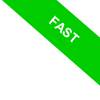
The callable() Function in Python
Let's explore a Python function called callable(). At first glance, this function might seem a bit puzzling, but it’s actually straightforward and quite handy once you get the hang of it.
callable(object)
The callable() function is a simple tool provided by Python to check if an object can be "called" like a function.
In other words, callable() lets you determine whether you can use parentheses () after an object to execute code.
How does it work?
When you invoke callable(obj), Python will return:
- True if the object obj can be called like a function.
- False if it cannot be called.
To clarify, let's look at some examples of objects that are considered "callable" in Python:
- Functions: Functions are naturally callable. If you have a function defined as def greet(), you can call it with greet(), so callable(greet) will return True.
- Methods: Methods are functions associated with an object and are also callable. If you have a method like object.method(), you can call it, making it callable.
- Classes: Classes in Python are callable as well. When you call a class, Python creates a new instance of that class. For instance, callable(MyClass) will return True.
- Objects with a __call__ method: You can make any object callable by implementing the special __call__ method in its class.
Now, let’s go through some practical examples.
Start by defining a simple function called greet().
def greet():
print("Hello, world!")
Next, check if the function is callable using callable().
The result is True because functions are inherently callable.
print(callable(greet))
True
Now, let’s see if a variable is callable.
x = 42
In this case, x is just a number; it’s not something you can "call", so callable(x) returns False.
Simply put, you can’t pass arguments to the variable using parentheses.
print(callable(x))
False
Let’s take a look at how this works with classes and objects.
Define the class Person.
class Person:
def __init__(self, name):
self.name = name
Every class is callable, as it’s used to instantiate objects.
Since Person is a class, it is callable because you can invoke it to create an instance.
print(callable(Person))
True
Now, create an instance of the Person class.
p = Person("Alice")
Then, check if the instance is callable.
In this case, the result is False because instances are not callable by default.
print(callable(p))
False
To make instances of a class callable, you need to define the __call__ method within the class.
class Person:
def __init__(self, name):
self.name = name
def __call__(self, second_name):
self.second_name = second_name
print(f"Hello, {self.second_name}! My name is {self.name}")
Now, create an instance of the Person class.
p = Person("Alice")
Then, check if the instance is callable.
In this case, the Person class has a __call__ method defined. This allows you to "call" an instance of the class as if it were a function. So, the result is True.
print(callable(p))
True
For example, if you call the instance and pass a string as an argument, Python will print a greeting message.
p("Mario")
Hello, Mario! My name is Alice
When should you use the callable() function?
Generally, the callable() function is extremely useful when you're working with functions and objects dynamically, especially when you're unsure if something you've received as input can be called like a function.
By using callable(), you can prevent errors and ensure your code only handles objects that can actually be executed as functions.