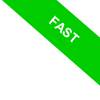
The built-in function issubclass()
In Python, the built-in issubclass() function serves a crucial role: it determines whether a given class is a subclass of another class or a tuple of classes. Here's the basic syntax:
issubclass(class1, class2)
This function accepts two arguments:
- class1 - the class you're investigating.
- class2 - either a single class or a tuple of classes against which you're comparing class1.
If class1 is a subclass of any class specified in class2, the function returns True. Otherwise, it yields False.
A word of caution: if the second argument isn't a class or a tuple of classes, the function throws a TypeError with the message "isinstance() arg 2 must be a class, type, or tuple of classes and types". It's essential to handle such exceptions, especially when the type of the object in the second argument might be ambiguous.
Let's delve into a practical example.
Start by defining a class
- class A:
- pass
Then, carve out a subclass from class A
- class B(A):
- pass
For the sake of comparison, introduce another class, C, unrelated to the first two
- class C:
- pass
To ascertain if class B is a derivative of class A
print(issubclass(B, A))
As expected, the output is True
since B originates from A.
True
However, when you evaluate if class C is a descendant of A
print(issubclass(C, A))
The function returns False, confirming C's independence from A
False
The versatility of issubclass() extends further. You can juxtapose a class against a tuple of classes.
The function will affirm True if the class is a descendant of at least one class in the tuple.
Consider a new subclass of B
- class D(B):
- pass
Now, determine if D is a progeny of either A or C:
print(issubclass(D, (A, C)))
While D doesn't directly descend from C, it's an offshoot of B, which itself is rooted in A.
Thus, the function confirms this lineage with True.
True
Lastly, it's worth noting that in Python's eyes, every class is inherently a subclass of itself.
So, when you compare a class to itself, the verdict is unsurprisingly True.
print(issubclass(A, A))
True