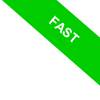
The __base__ attribute in Python
In Python, the `__base__` attribute of a class indicates the name of the parent class from which a particular subclass directly inherits.
__base__
This attribute is useful when working with inheritance in Python, allowing you to examine the class hierarchy.
Understanding the class hierarchy is crucial for leveraging inheritance and polymorphism in Python effectively.
Inheritance allows you to create new classes that inherit properties and methods from existing classes, promoting code reuse and a more organized structure.
Let's look at some practical examples to understand how `__base__` works.
Define two classes, `Dog` and `Animal`.
class Animal:
def speak(self):
pass
class Dog(Animal):
def speak(self):
return "Woof woof!"
In this example, `Dog` inherits from `Animal`.
By using the `Dog.__base__` attribute, you can see that the direct base class of `Dog` is `Animal`.
print(Dog.__base__)
<class '__main__.Animal'>
Let's look at another example with a slightly more complex hierarchy.
class LivingBeing:
def exist(self):
return "I exist!"
class Animal(LivingBeing):
def speak(self):
pass
class Dog(Animal):
def speak(self):
return "Woof woof!"
In this case, the class `Dog` inherits from `Animal`, which in turn inherits from `LivingBeing`.
Using the `__base__` attribute, you can trace this hierarchy step by step.
print(Animal.__base__)
<class '__main__.LivingBeing'>
And naturally,
print(Dog.__base__)
<class '__main__.Animal'>
Alternatively, you can create a loop to traverse the classes from the child class to the parent class.
def print_hierarchy(cls):
while cls:
print(cls)
cls = cls.__base__
print_hierarchy(Dog)
This small iterative script prints the class hierarchy starting from `Dog` until it reaches the top level, which is usually `object` in Python.
<class '__main__.Dog'>
<class '__main__.Animal'>
<class '__main__.LivingBeing'>
<class 'object'>
In conclusion, the `__base__` attribute allows you to explore the class hierarchy in Python and gain a better understanding of class inheritance. It is particularly useful when working with frameworks or complex libraries that make extensive use of inheritance.