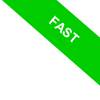
Overriding and Overwriting Methods in Python
In Python's object-oriented programming (OOP), overriding and overwriting are two fundamental concepts. Although they are often confused, they play distinct roles in how methods are inherited from a base class.
- Overriding
The derived class inherits and extends the behavior of a method from the base class. - Overwriting
The derived class completely replaces the behavior of a method from the base class.
These concepts are essential for designing classes that make effective use of inheritance, as they allow you to reuse code and customize the behavior of derived classes. Let's explore them in detail.
Method Overriding
Overriding occurs when a derived class (or subclass) inherits and expands on a method defined in the base class (or superclass).
This allows the subclass to customize or extend the behavior of the method inherited from the superclass.
For example, consider this base class called `Animal`:
class Animal:
def sound(self):
print('The sound this animal makes is: ')
Next, define a subclass called `Dog` that inherits methods and attributes from the `Animal` class.
class Dog(Animal):
def sound(self):
super().sound()
return "Woof Woof!"
The derived class calls the base class's sound() method using the super() function and adds an additional line of code.
Now, create an instance of the Dog class.
dog = Dog()
Call the sound() method on the instance.
print(dog.sound())
The sound this animal makes is:
Woof Woof!
The instance executes both the base class `Animal`'s sound() method and the additional code in the `Dog` class.
Method Overwriting
Overwriting involves completely replacing a method from the base class.
This happens when a derived class (or subclass) provides its own implementation of a method already defined in its base class (or superclass), thus replacing the original method without calling it.
For example, imagine you have a base class called `Animal` with a method called `sound`:
class Animal:
def sound(self):
print('The sound this animal makes is: ')
Then create a subclass called `Dog` that inherits methods and attributes from the `Animal` class.
class Dog(Animal):
def sound(self):
return "Woof Woof!"
In this case, there is no call to super() in the sound() method. Therefore, the `Dog` class completely overwrites the sound() method of the parent class.
Now, create an instance of the Dog class.
dog = Dog()
Call the sound() method on the instance.
print(dog.sound())
Woof Woof!
As you can see, the `Dog` class has its own customized behavior for the sound() method, which replaces that of the base `Animal` class.
In this scenario, the original definition of the sound() method is completely ignored because the new one overwrites it.
I hope this guide has helped clarify the concepts of overriding and overwriting.