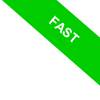
Modifying Instance Attributes in Python
This guide delves into the different techniques for altering instance attributes in a Python class, illustrated with practical examples.
What are instance attributes? Instance attributes are variables that belong to each specific instance of a class. They are usually defined within the class methods, particularly in the `__init__` method, which acts as the class constructor.
Modifying instance attributes is a routine yet crucial aspect of object-oriented programming in Python.
Let's start with a practical example.
Define a class called 'Car'.
class Car:
def __init__(self, color, doors):
self.color = color
self.doors = doors
This class incorporates two instance attributes: 'color' and 'doors'.
Create two instances of this class to see these attributes in action.
myCar1=Car('white', 5)
myCar2=Car('black', 4)
Each instance has uniquely assigned values to its attributes.
For example, in myCar1, the 'color' attribute is 'white', while in myCar2, it is 'black'.
print(myCar1.color)
white
There are multiple strategies to modify instance attributes: direct editing, using the setattr function, employing setter methods, or manipulating the instance's dictionary. Let's examine these methods.
Directly Modifying Instance Attributes
Direct modification is the simplest and most straightforward method to alter an instance attribute's value.
For instance, to change the 'color' attribute of myCar1, you could simply write:
myCar1.color = "blue"
This update changes the value assigned to the 'color' attribute.
print(myCar1.color)
blue
Using the setattr() Function
In Python, the setattr() function provides another way to set a new value for an instance attribute.
For example, to update the 'color' attribute of myCar1, you could use:
setattr(myCar1, 'color', 'green')
The new value replaces the previous one.
print(myCar1.color)
green
This method is especially useful for dynamically modifying an attribute based on run-time conditions or external data inputs.
Implementing Setter Methods
To ensure better encapsulation and control over attribute modification, it is advisable to use setter methods. These methods allow you to include validation logic before an attribute's value is altered.
Consider a 'Car' class with a setter method for 'color':
class Car:
def __init__(self, color, doors):
self._color = color
self._doors = doors
def set_color(self, color):
if color not in ["red", "blue", "green"]:
& nbsp; raise ValueError("Invalid color")
self._color = color
def get_color(self):
return self._color
def get_doors(self):
return self._doors
myCar1=Car('white', 5)
Here, the 'set_color' method checks if the new color is among the allowed options. If not, it raises a ValueError.
print(myCar1.get_color())
green
Setter and getter methods not only enhance the security and robustness of your code but also increase its maintainability, particularly in complex projects or collaborative environments.
Employing setter and getter methods can help maintain a higher level of control over the access and modification of attributes. Even though private attributes, marked with an underscore (e.g., `_color`), are just a convention in Python, they signify that these attributes should ideally not be accessed directly from outside the class.
The Instance Attribute Dictionary
Modifying an attribute directly through the instance's attribute dictionary, '__dict__', is another viable approach.
For instance, to view and then alter the 'color' attribute in myCar1's attribute dictionary:
print(myCar1.__dict__)
{'color': 'white', 'doors': 5}
To change the 'color' attribute through the dictionary, execute:
myCar1.__dict__['color'] = "blue"
The attribute 'color' is now updated to 'blue'.
print(myCar1.color)
blue
This Python feature facilitates direct access and manipulation of an object's attribute dictionary, useful in scenarios involving dynamic class construction or runtime behavior modification.
However, this method should be used cautiously, as it can obscure code clarity and make it more challenging to maintain. Direct manipulation via the `__dict__` may also circumvent any encapsulation and validation logic that might be implemented in setter methods or other parts of the class. This can lead to potential bugs, errors, or unexpected behavior.
Ultimately, while direct dictionary manipulation is technically feasible and can be helpful under specific circumstances, it's generally better to use the setattr() and getattr() functions for setting and retrieving instance attributes in a controlled manner.
Adhering to these practices ensures that instance attributes are managed effectively and securely, maximizing the benefits of object-oriented programming in Python.