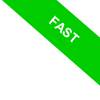
The __getattr__() Method in Python
In Python, the `__getattr__()` method is a special function that gets automatically triggered when you try to access an attribute that hasn't been defined in an object.
object.__getattr__()
This method handles access to attributes that don't exist in the object.
It allows you to catch these requests and provide a default value or some alternative behavior.
In other words, when you try to access something that isn't directly available in the object, Python says, "Hold on! Before failing, let’s see if there’s another way to handle this."
Let’s go through an example.
Create a class called 'Person'.
class Person:
def __init__(self, name):
self.name = name
Then, create an instance of the class.
p = Person("Luca")
If you try to access the 'name' attribute of the object, it will return the value that was assigned to it.
print(p.name)
Luca
Now, try accessing a non-existent attribute, like 'p.age'.
print(p.age)
Since Python can't find the 'age' attribute in the object, it raises an error.
Traceback (most recent call last):
File "/home/main.py", line 8, in <module>
print(p.age)
AttributeError: 'Person' object has no attribute 'age'
To handle this more gracefully, you can define the '__getattr__()' method in the 'Person' class.
This method will be called automatically whenever you try to access a non-existent attribute.
class Person:
def __init__(self, name):
self.name = name
def __getattr__(self, attr):
return f"The attribute '{attr}' does not exist."
Now, create an instance of the class, for example, the object 'p'.
p = Person("Luca")
Then, try to access a non-existent attribute on the object 'p'.
print(p.age)
Since Python can't find the 'age' attribute, it automatically calls the '__getattr__()' method, which returns a message instead of an error.
The attribute 'age' does not exist
By doing this, the program returns a meaningful response even when an attribute doesn’t exist, rather than throwing an error.
Difference Between `__getattr__()` and `__getattribute__()`
Python also has another similar method called `__getattribute__()`. Be careful not to confuse them!
- The `__getattr__()` method is only called when the attribute does not exist.
- The `__getattribute__()` method, on the other hand, is called every single time you try to access any attribute, whether it exists or not.
In most cases, `__getattr__()` is more than sufficient. `__getattribute__()` can be more tricky since it can interfere with normal attribute access, so it should be used with care.
Lazy loading
The '__getattr__()' method can be leveraged to load attributes only when they're accessed, which helps reduce initial overhead or manage resources that take longer to process.
Here’s a practical example of lazy loading in action:
class Person:
def __init__(self, name):
self.name = name
self._age = None # The age attribute starts out undefined
def __getattr__(self, name):
if name == 'age':
if self._age is None:
print(f"The '{name}' attribute hasn't been initialized. Please enter your age:")
# Prompt the user to input their age
var = int(input("Enter your age: "))
super().__setattr__('_age', var)
return self._age
In this class, the person's age is not needed at the time of object initialization.
If the user tries to access the 'age' attribute before it’s defined, they’ll be prompted to enter it through input().
Here’s how you can create an instance of the class:
p = Person("Anna")
Now, attempt to access the 'age' attribute:
print(p.age)
Since it hasn’t been defined yet, Python will prompt you to provide the person's age.
The 'age' attribute hasn't been initialized. Please enter your age:
Enter your age:
In summary, the `__getattr__()` method acts as a fallback mechanism that lets you gracefully handle cases where attributes don't exist.
When used wisely, it can simplify your code by eliminating the need for multiple `if` statements and existence checks.