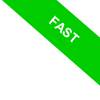
The __call__ Special Method in Python
Today, we're going to explore an intriguing and powerful feature of Python programming: the __call__ special method.
What is the `__call__` method? This method allows you to do something quite unusual yet incredibly useful: it turns an instance of a class into a "callable" object, just like a function.
In Python, all functions are callable objects, which means you can invoke them using parentheses `()`.
For example, let’s define a simple function:
def greet():
print("Hello!")
You can call the function like this:
greet()
This invokes the function and prints:
"Hello!"
Now, imagine being able to do the same with an instance of a class—essentially, with a class object. This is made possible by the `__call__` method.
For example, let’s define a class called "Rectangle."
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
Then, create an instance of the class named "rect1."
rect1 = Rectangle(5,4)
This object stores the width (5) and height (4) of the rectangle you’ve specified.
print(rect1.width)
5
However, it’s not a callable object because, by default, instances of a class are not callable. They simply store data.
So, you can't invoke the instance as if it were a function by passing arguments in parentheses.
print(callable(rect1))
False
If you try, Python will raise an exception:
print(rect1(2))
Traceback (most recent call last):
File "/home/main.py", line 12, in <module>
print(rect1(2))
TypeError: 'Rectangle' object is not callable
To make instances of the class callable, you need to define the special method `__call__`.
When you define `__call__` within a class, you’re essentially telling Python what should happen when an instance of that class is "called" like a function.
For example, let’s modify the "Rectangle" class as follows:
class Rectangle:
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
def __call__(self, factor):
return self.area() * factor
Now, create an instance of the class:
rect1 = Rectangle(5,4)
You can now "call" the object as if it were a function.
print(callable(rect1))
True
When Python detects parentheses after the instance, it automatically triggers the __call__ method.
print(rect1(2))
In this example, the __call__ method calculates the area of the rectangle and multiplies it by the number passed as an argument, in this case, 2.
The result is 4x5x2=40
40
In summary, the `__call__` method in Python allows instances to behave like functions while retaining all the benefits of an object, such as internal state and associated methods.
Why use `__call__`? The `__call__` method can be incredibly useful in a variety of situations. For example, you can create objects that behave like custom functions but maintain state or other important information. It’s especially handy when you want to combine the simplicity of a function with the complexity of an object.
So, the next time you’re designing a class and think it could be used like a function, consider adding `__call__` to make it even more intuitive and versatile.