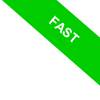
Removing a Class Attribute in Python
To remove a class attribute, simply use the `del` command followed by the class and attribute name.
del attributeName
This command erases the class attribute from the class's dictionary, rendering it inaccessible via the class itself or its instances unless it has been overridden on an instance level.
The deletion of a class attribute in Python can profoundly affect the behavior of its instances, as it is shared across all of them.
Exercise caution when deleting a class attribute. Class attributes are variables that are defined within the class scope but outside its methods, and are shared among all instances. Deleting one impacts all instances that have been created from that class, rendering the attribute invisible to any existing instance.
Here's a practical example:
Define a straightforward class.
class Car:
wheels = 4 # Class attribute
This class includes a class attribute named 'wheels'.
Create several instances using the 'Car' class:
myCar1 = Car
myCar2 = Car
If you query the class attribute on either instance, myCar1 or myCar2, it returns a value of 4.
print(myCar1.wheels)
4
You can also access the class attribute directly from the 'Car' class:
print(Car.wheels)
4
Now, delete the 'wheels' class attribute using the del instruction.
del Car.wheels
After you delete the 'wheels' attribute, attempting to access `Car.wheels` will cause Python to raise an `AttributeError` because the attribute no longer exists in the class dictionary.
print(Car.wheels)
AttributeError: type object 'Car' has no attribute 'wheels'
The same error occurs if you attempt to access the class attribute through any existing instance of the class.
For instance, attempting to access myCar1.wheels results in Python raising an `AttributeError`.
print(myCar1.wheels)
AttributeError: type object 'Car' has no attribute 'wheels'
This occurs because the class attribute is shared among all instances.
Once a class attribute is deleted, it cannot be accessed either from the class itself or from any of its instances unless it was explicitly overridden in individual instances.
How to Override a Class Attribute with an Instance Attribute: If the functionality of your instances depends on a class attribute, consider introducing an equivalent instance attribute before removing the class attribute. For example, here’s how you could structure such a class:
class Car:
wheels = 4 # class attribute
def __init__(self, wheels=None):
if wheels is not None:
self.wheels = wheels # instance attribute
myCar1=Car(4)
myCar2=Car()
del Car.wheels
print(myCar1.wheels)
4
In this case, the constructor assesses whether the 'wheels' parameter has been provided during instance creation. If specified, the instance will substitute the class attribute 'wheels' with a personalized instance attribute. Without the parameter, the instance will continue referring to the class attribute. Therefore, instances like myCar1, which have replaced the class attribute with a personalized instance attribute, will retain this value even after the class attribute 'wheels' is deleted. Conversely, other instances like myCar2 will lose access to the class attribute.
Ultimately, removing a class attribute should not be taken lightly, as it impacts all instances of the class.
Before proceeding, consider the potential impact and adjust your code to manage instance behavior more effectively, ensuring robustness and preventing unexpected errors.