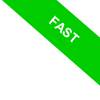
The __getattribute__() Method in Python
In Python, when you access an attribute of an object, the language doesn’t just grab it directly. Instead, it first passes through __getattribute__(), which is responsible for locating that attribute.
object.__getattribute__()
If the attribute exists, __getattribute__() will return it. If not, it raises an AttributeError.
So, every time you access an attribute, it always goes through this method first.
What’s the difference between __getattribute__() and __getattr__()? The __getattribute__() method is invoked every time you try to access an attribute. In contrast, the __getattr__() method is only called if the attribute isn’t found in the object.
Here’s how it works.
Let’s say you have this simple class:
class Student:
def __init__(self, name, age):
self.name = name
self.age = age
Create an instance of the class:
s = Student('Anna', 22)
Now try accessing the 'name' attribute:
print(s.name)
When you do this, Python is actually calling s.__getattribute__('name') behind the scenes to retrieve the value 'Anna'.
Anna
You could achieve the same result by calling the __getattribute__() method directly:
print(s.__getattribute__('name'))
Anna
So far, everything should make sense, but it might seem a little redundant.
Why would you use __getattribute__()?
The real benefit of __getattribute__() is that it lets you customize how attribute lookups are handled for an object.
In other words, you can override __getattribute__() to change the behavior of your class whenever someone accesses its attributes.
Here’s a practical example:
class Student:
def __init__(self, name, age):
self.name = name
self.age = age
def __getattribute__(self, attr):
print(f"Looking for attribute {attr}")
return super().__getattribute__(attr)
This simple code prints a custom message every time an attribute is accessed.
For example, create an instance of the class:
s = Student('Anna', 22)
Then try accessing an attribute:
print(s.name)
See? Before returning the 'name' value, Python prints that message. This happens because you’ve overridden __getattribute__().
Looking for attribute name
Anna
This example is simple, but you could modify it to perform more complex operations, such as protecting access to certain attributes or adding custom logic before returning values.
Warning: Avoid infinite loops. If you try to access an attribute like self.name directly inside __getattribute__(), you’ll create an infinite loop! This happens because you’d be calling __getattribute__() again to retrieve the attribute, and so on, forever. The solution? Always use super().__getattribute__(attr) to avoid the loop and call the original method.
When should you use __getattribute__()?
Think of __getattribute__() as a gatekeeper that monitors every access to an object’s attributes. However, it requires careful use to avoid potential issues.
In most cases, it’s more common to use __getattr__(), which is only called when an attribute is not found.
However, if you need full control over every attribute access, __getattribute__() is the right tool for the job, since it’s invoked regardless of whether the attribute exists or not.
But beware! Since __getattribute__() is so fundamental to how objects work, modifying it carelessly can cause big problems. If you’re not careful, you might end up with an infinite loop, because every access to an attribute—even internal ones—goes through __getattribute__().