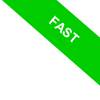
Super Classes in Python
Today we're going to discuss super classes in Python. These classes allow you to call methods from a base class, making inheritance and code reuse in derived classes much easier.
super()
You can create a "super class" using the super() function, which is crucial for handling class inheritance. This function lets you create a new derived class that inherits attributes and methods from an existing base class.
The super() function returns a temporary object of the parent class, allowing you to call its methods directly from the derived class.
Why use super()? It's especially useful in an inheritance hierarchy where you might want to extend or alter the behavior of a base class method in a derived class. For instance, if you change a method in the base class, those changes automatically apply to the derived classes. Additionally, you can call a base class method without explicitly naming the base class, which helps keep the code flexible and reduces dependency on specific class names.
Let's look at a practical example.
Imagine you have a base class `Animal` and a derived class `Dog`, and you want the `Dog` class to extend some functionalities of the `Animal` class. You can do this using the super() function.
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
raise NotImplementedError("This method must be implemented by the subclass")
class Dog(Animal):
def __init__(self, name, breed):
super().__init__(name) # Calls the constructor of the base class
self.breed = breed
def speak(self):
return f"{self.name} says: Woof Woof!"
In this example, the base class `Animal` defines a constructor (`__init__`) that takes the animal's name and a `speak` method that raises an exception. This method is intended to be overridden in derived classes.
The derived class `Dog` defines a constructor that takes both the dog's name and breed, using the `super().__init__(name)` function to initialize the `name` attribute with the base class's constructor. Additionally, it overrides the `speak` method to provide a specific implementation for dogs.
Note that you don't need to specify the base class name in the super() function because Python automatically derives it from the object's '__base__' attribute. This simplification was introduced in Python3. If you're using an earlier version, Python2, you need to specify the base class name in the super() function's parentheses.
For instance, create an instance of the Dog class.
my_dog = Dog("Fido", "Labrador")
Now, call the speak() method from the 'my_dog' instance.
print(my_dog.speak())
Fido says: Woof Woof!
The object has inherited the 'name' attribute from the base class and overridden the speak() method.
In this example, you used the super() function in the 'Dog' class to call the 'name' attribute from the base class ('Animal') constructor.
class Dog(Animal):
def __init__(self, name, breed):
super().__init__(name) # Calls the constructor of the base class
self.breed = breed
def speak(self):
return f"{self.name} says: Woof Woof!"
You could achieve the same result without using the super() function by explicitly naming the parent class. In this case, you would need to specify 'Animal'. However, this approach is less flexible and can be more challenging to maintain, especially in more complex projects or when using multiple inheritance.
class Dog(Animal):
def __init__(self, name, breed):
Animal.__init__(name) # Calls the constructor of the base class
self.breed = breed
def speak(self):
return f"{self.name} says: Woof Woof!"
Multiple Inheritance with Super Classes
The use of `super()` becomes even more important in scenarios involving multiple inheritance.
Let's look at a more complex example with multiple inheritance:
class A:
def method(self):
print("Method from A")
class B(A):
def method(self):
print("Method from B")
super().method() # Calls method from A
class C(A):
def method(self):
print("Method from C")
super().method() # Calls method from A
class D(B, C):
def method(self):
print("Method from D")
super().method() # Calls method from B and then from C
In this example, class `A` defines a simple method that prints a string.
Classes `B` and `C` inherit from `A` and override the `method`, calling the base class method using the super() function.
Class `D` inherits from both `B` and `C`. In this case, super().method() follows the Method Resolution Order (MRO), calling `method` from `B`, then `method` from `C`, and so on.
For instance, create an instance of class D
d = D()
Then, call the method from the instance.
d.method()
Python follows the MRO to determine the call order, and the program output will be:
Method from D
Method from B
Method from C
Method from A
In conclusion, using `super()` is essential for effectively managing inheritance in Python, as it makes the code more maintainable and flexible.
Understanding how and when to use `super()` will enable you to write more robust and reusable classes.