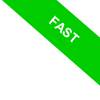
The __class__ Attribute in Python Objects
In Python, every object is equipped with a special attribute called `__class__`, which identifies the class to which the object belongs.
object.__class__
This attribute is not just a formal part of the object; it plays a crucial role by holding a reference to the object’s type, or class.
This feature is invaluable for introspection, enabling developers to retrieve information about the type or class of an object instance.
As an alternative, Python offers the type() function, allowing you to achieve similar results. For instance, type(obj) yields the same outcome as obj.__class__.
Consider a class named `Automobile`:
class Automobile:
def __init__(self, make, model):
self.make = make
self.model = model
Create an instance of this class, let's call it `my_car`:
my_car = Automobile("Tesla", "Model S")
You can then use `my_car.__class__` to directly access its class, `Automobile`.
print(my_car.__class__)
The system fetches the __class__ attribute of the object and returns `Automobile`.
<class '__main__.Automobile'>
The __class__ attribute also allows you to determine the type of an object.
For example, create a list:
obj = [1, 2, 3]
Querying the __class__ attribute of the newly created "obj" will reveal its nature.
print(obj.__class__)
Python will return 'list' as the type of the object.
<class 'list'>
Furthermore, the __class__ attribute can be used to access class attributes.
class MyClass:
class_variable = 10
instance = MyClass()
print(instance.__class__.class_variable)
10
It's also possible to use __class__ to instantiate new objects from the same class as an existing object, which is particularly handy in generic copying or cloning operations.
instance2 = instance.__class__()
This method creates another object "instance2" from the same class as "instance".
print(instance2.__class__)
<class '__main__.MyClass'>
Typically, the __class__ attribute is a powerful tool for debugging and introspection, allowing dynamic exploration of an object's properties and methods during the runtime of a script.
It also facilitates the implementation of polymorphism and dynamic type manipulation.
Nevertheless, it's wise to use `__class__` judiciously, as indiscriminate access to an object’s internal metadata can obscure your code and make it harder to maintain. It can also lead to unpredictable behavior if misused. In most instances, the type() function is a safer, more idiomatic choice . It is immediately recognizable, avoids delving into the object itself, and is compatible across all versions of Python.
class MyClass:
class_variable = 10
instance = MyClass()
print(type(instance))
<class '__main__.MyClass'>
The type() function is a familiar sight in Python scripts, offering a simple, universally applicable way to determine an object’s class without internal access.