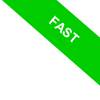
Python's getattr() Function
The getattr() function in Python serves a dynamic role in accessing attribute values of an object. It's an elegant solution for retrieving properties when the structure of an object is not static or fully known ahead of time.
getattr(object, name[, default])
Function parameters are straightforward:
- object: The target object from which to fetch the attribute.
- name: The attribute name as a string.
- default (optional): A fallback value if the attribute is missing. Omitting this parameter causes a raised "AttributeError" if the attribute is absent.
By using getattr(), you're directly tapping into the object's attribute, fetching its assigned value seamlessly.
This function shines in scenarios where you're dealing with objects of varying structures, allowing for flexible attribute access. It gracefully handles non-existent attributes by permitting a default return value, circumventing potential errors.
Let's dive into a real-world example:
Imagine a "Person" class with attributes for "name" and "age".
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
Create an instance of this class:
person = Person("Mario", 30)
This instance now holds "Mario" as the name and 30 as the age.
Accessing these attributes is streamlined with getattr():
For the "name" attribute, "Mario" is effortlessly retrieved and displayed.
person_name = getattr(person, "name")
print(person_name)
Mario
Similarly, accessing the "age" attribute yields 30.
person_age = getattr(person, "age")
print(person_age)
30
An attempt to fetch a non-existent "hobby" attribute demonstrates getattr()'s robust error handling, raising an "AttributeError".
person_hobby = getattr(person, "hobby")
Traceback (most recent call last):
File "/home/main.py", line 14, in <module>
person_hobby = getattr(person, "hobby")
AttributeError: 'Person' object has no attribute 'hobby'
Setting a default value avoids such errors, demonstrating getattr()'s flexibility.
Accessing a Non-existent Attribute with a Default Value
Specifying a default for missing attributes, such as a "Not specified" for "hobby", showcases graceful error handling.
person_hobby = getattr(person, "hobby", "Not specified")
print(person_hobby)
Instead of an error, getattr() returns the designated fallback, ensuring smooth execution.
"Not specified"
This technique significantly enhances program resilience by elegantly managing the absence of expected attributes.