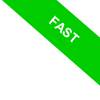
The __str__ Method in Python Classes
Today, we'll explore the __str__ method in Python classes. This method lets you define how an object should be represented as a string.
__str__
The `__str__` method is called when you use the str() function or the print() function on an object.
It's one of the special methods in Python, also known as "dunder" methods (short for "double underscore"), which provide special functionality to objects.
What's its purpose? Imagine you have a complex class and want to print a readable representation of its state. By default, Python uses a standard representation that usually isn't very informative. By defining the `__str__` method, you can customize this representation to make it clearer.
Let's look at a practical example to understand how it works.
Suppose you have a `Persona` class:
class Persona:
def __init__(self, nome, eta):
self.nome = nome
self.eta = eta
Create an instance of Persona:
persona = Persona("Alice", 30)
If you print the object, Python returns the object's reference, which isn't very helpful.
print(persona)
<__main__.Persona object at 0x7f4748338310>
Now, add the `__str__` method to the class:
class Persona:
def __init__(self, nome, eta):
self.nome = nome
self.eta = eta
def __str__(self):
return f"Persona(nome={self.nome}, eta={self.eta})"
Create a new instance of Persona:
persona = Persona("Alice", 30)
When you print the object, Python calls the `__str__` method, displaying the person's name and age. Much better, right?
print(persona)
Persona(nome=Alice, eta=30)
Of course, you can customize the return message and the information according to your needs.
Always remember that the `__str__` method must return a string (`str`).
Another similar method is __repr__. The main difference is that `__repr__` is designed to provide a more detailed and precise representation of the object, while `__str__` is meant for a more user-friendly representation.