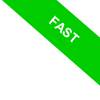
The super() Function in Python
The super() function in Python allows you to access attributes and methods of a parent class (superclass) within a derived class (subclass).
super()
This function is especially useful when working with inheritance, as it enables you to extend or modify the behavior of parent classes.
Why use super()? Imagine having a set of classes that share some common functionality. Instead of duplicating the same code in each class, you can place that code in a base class and extend it in the subclasses. The super() function lets you refer to these methods and attributes of the base class without rewriting them.
Let’s look at a simple example with classes representing vehicles.
class Vehicle:
def __init__(self, make, model):
self.make = make
self.model = model
def description(self):
return f"{self.make} {self.model}"
class Car(Vehicle):
def __init__(self, make, model, type):
super().__init__(make, model)
self.type = type
def description(self):
return f"{super().description()} - Type: {self.type}"
In this code, the `Vehicle` class is our base class with a constructor (`__init__`) that initializes the vehicle’s make and model. It also has a `description` method that returns a string with the make and model.
The `Car` class, on the other hand, is a subclass of `Vehicle`.
- In its constructor, it calls the superclass constructor (`super().__init__(make, model)`) to initialize the make and model, then adds a specific attribute `type`.
- The `description` method in the `Car` class extends the superclass’s `description` method. It uses `super().description()` to get the base description and adds specific information about the car.
In this way, when we create an instance of `Car` and call `description`, we get a string that combines the information from the base class and the subclass.
For example, create an instance of the `Car` class.
car = Car("Toyota", "Corolla", "Sedan")
Now call the `description` method on the instance.
print(car.description())
Toyota Corolla - Type: Sedan
Note that you could achieve the same results by directly specifying the name of the parent class `Vehicle` instead of using the super() function.
class Vehicle:
def __init__(self, make, model):
self.make = make
self.model = model
def description(self):
return f"{self.make} {self.model}"
class Car(Vehicle):
def __init__(self, make, model, type):
Vehicle.__init__(self, make, model)
self.type = type
def description(self):
return f"{Vehicle.description(self)} - Type: {self.type}"
However, the super() function offers advantages in terms of maintainability, compatibility with multiple inheritance, and support for polymorphism. For a deeper dive, read our tutorial on multiple inheritance in super classes.
So, even though it's possible to achieve the same results without super(), its use makes the code more robust and flexible.
What are the advantages of the super() function?
The super() function allows you to reuse code, avoiding duplication and making maintenance easier.
Additionally, it enables you to extend the functionality of base classes without modifying them directly.
It facilitates the use of the Liskov substitution principle, one of the SOLID principles of object-oriented programming, ensuring that subclasses can replace base classes without breaking the program’s behavior.
So, the next time you're working with classes in Python, remember to leverage `super()` to get the most out of object-oriented programming.