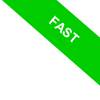
Instances in a Python Class
In Python, a class instance is a specific object created based on the structure and blueprint defined by its class.
Think of a class as a blueprint for crafting objects that not only share a similar structure but also exhibit identical behavior.
Creating an object from a class means you are generating an instance of that class.
In Python, to instantiate a class, simply use the class name followed by parentheses.
For instance, if you have a class named `Car`, you can create an instance with `my_car = Car()`.
#class definition
class Car:
pass
# Creating a Car instance
my_car = Car()
Each instance can possess attributes (variables) known as properties that store its state.
Attributes can be pre-defined in the class or added dynamically to an instance as needed.
For example, if a `Car` has a `color` attribute, you can change it by typing `my_car.color = 'red'` and access it by simply using `my_car.color`.
#class definition
class Car:
# class attribute
color= None
# Creating a Car instance
my_car = Car()
# Assign a value to the "color" attribute of the instance my_car
my_car.color = 'red'
# Print the attribute's content
print(my_car.color)
red
You can even dynamically append an attribute not originally in the class to an instance.
In such cases, the attribute is added solely to the specific object.
#class definition
class Car:
# class attribute
color= None
# Creating a Car instance
my_car = Car()
# Assign a value to the "year" attribute of the instance my_car
my_car.year = '2015'
# Print the attribute's content
print(my_car.year)
2015
Instances also feature methods, which are functions embedded within the class.
These methods dictate how objects of the class behave, can alter the state of the instance, or perform calculations using the attributes of the instance.
For instance, if the class `Car` includes a method named `describe()`, you can activate it by typing `my_car.describe()`.
#class definition
class Car:
# class attribute (property)
color= None
# executable attribute (method)
def describe(self):
print(f"A car of color {self.color}")
# Creating a Car instance
my_car = Car()
# Assign a value to the "color" attribute of the instance my_car
my_car.color = 'red'
# Call the describe() method to print the color of the car
my_car.describe()
Calling the describe() method accesses the "color" property of "my_car" and outputs the following:
A car of color red
Within class methods, the `self` keyword references the instance on which the method is being invoked, allowing seamless access to the instance’s attributes and methods.
The __init__ Method
A class may include a special method known as `__init__()` that automatically executes when an instance is created, typically used to set up initial attribute values.
Example
Add an initializer to the `Car` class to establish the `color` attribute upon creation:
class Car:
# initialization
def __init__(self, color):
self.color = color
# method
def describe(self):
return f"A car of color {self.color}"
# Create a Car instance specifying the color
my_car = Car("red")
# Invoke the "describe" method
print(my_car.describe())
A car of color red
This demonstrates how a class acts as a framework for generating objects (`my_car`) endowed with specific attributes and predefined behaviors.