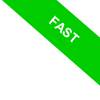
Object Composition in Python
Today, we're going to discuss object composition in the Python programming language.
What is composition? Composition is a key concept in object-oriented programming (OOP) that allows you to create complex objects by combining simpler ones. This concept is highly applicable to Python, where an object's attributes can reference other objects.
It centers on the idea of building a composite object that uses objects from other classes to provide the desired functionality.
With composition, each class has a single responsibility, making the code easier to understand.
This approach lets you build a complex data structure using simpler, reusable components.
What are the benefits? You can reuse a class to build other classes without repeating the same code. In other words, you can extend a class by adding new methods or attributes without modifying the original class.
Let me provide a practical example.
Imagine you need to work with geometric shapes (e.g., circle, square, rectangle, etc.).
Before creating the shape classes, it's useful to create a base class 'Point' to represent a generic point in a plane.
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
def __str__(self):
return f"({self.x}, {self.y})"
This class is straightforward and represents a point (x;y) with coordinates x and y.
Now, create a `Circle` class that uses a `Point` object to indicate the center of the circle.
class Circle:
def __init__(self, center, radius):
self.center = center
self.radius = radius
def area(self):
import math
return math.pi * (self.radius ** 2)
def circumference(self):
import math
return 2 * math.pi * self.radius
def __str__(self):
return f"Circle with center at {self.center} and radius {self.radius}"
In this class, `center` is a `Point` object representing the circle's center, while `radius` is a number.
The class includes three methods that perform specific calculations:
- The `area` method calculates the circle's area.
- The `circumference` method calculates the circle's circumference.
- The `__str__` method provides a readable representation of the circle.
Now, let's put everything together to see how it works.
Create an instance of the 'Point' class, specifying the coordinates (3,4) of the plane, i.e., x=3 and y=4.
center = Point(3, 4)
Then create an instance of the 'Circle' class using the point as the center and a radius of 5.
circle = Circle(center, 5)
The new 'circle' object is composed of the 'center' object and a number representing the radius.
At this point, you can call the methods to obtain various details about the geometric shape.
For example, you can display the circle's details.
print(circle)
Circle with center at (3, 4) and radius 5
You can calculate the circle's area.
print(circle.area())
78.53981633974483
You can also calculate the circumference.
print(circle.circumference())
31.41592653589793
In this example, you've seen how to use composition to create a circle starting from a simple point on the Cartesian plane.
This approach makes your code modular and easier to understand and maintain.
Note that you can use the same 'Point' object to define classes for other geometric shapes, such as rectangles, squares, etc. Reusability is one of the main advantages of object composition in Python code.